Subgrid
It's important to mention that these examples are in no way exhaustive (though they may be exhausting). Hopefully these will inspire you to see the potential, learn more, and try new things.
There are a lot of things you can do with grid
and, because these examples are specific to subgrid
, we won't go into all the ways you can use grid here. Instead we'll briefly look at a standard grid example and then see the difference between a nested grid and subgrid.
Standard grid example
This is a pretty standard use case for grid
: a uniform grid pattern layout. Each grid item is the same width and they are filled to the container size. In this case, we are using a minimum width of 250px and otherwise 1fr. Adjust your browser window larger/narrower to see how it responds to different sizes.
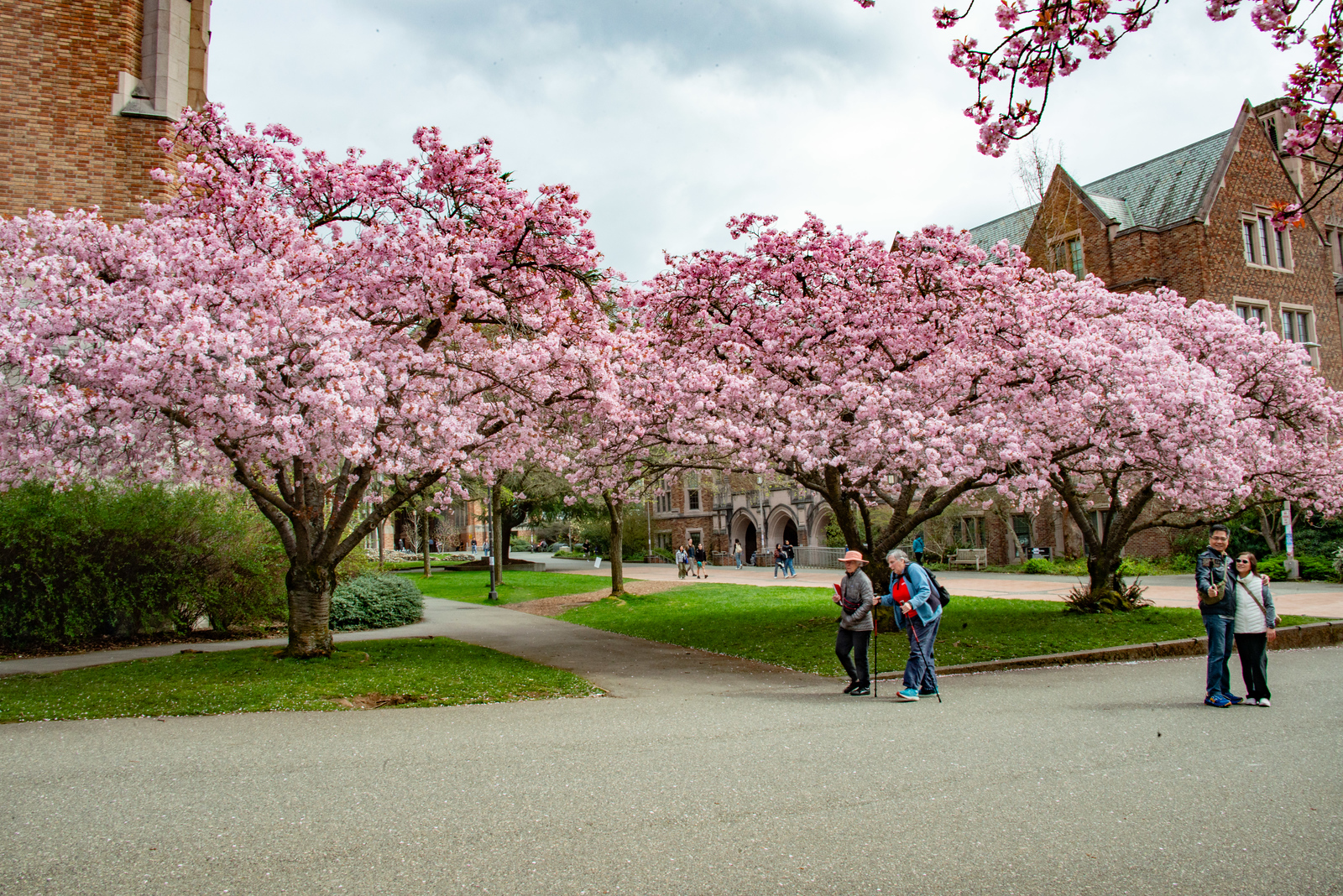
Sample grid item
Cupcake ipsum dolor sit amet. Liquorice bear claw tiramisu sweet shortbread.
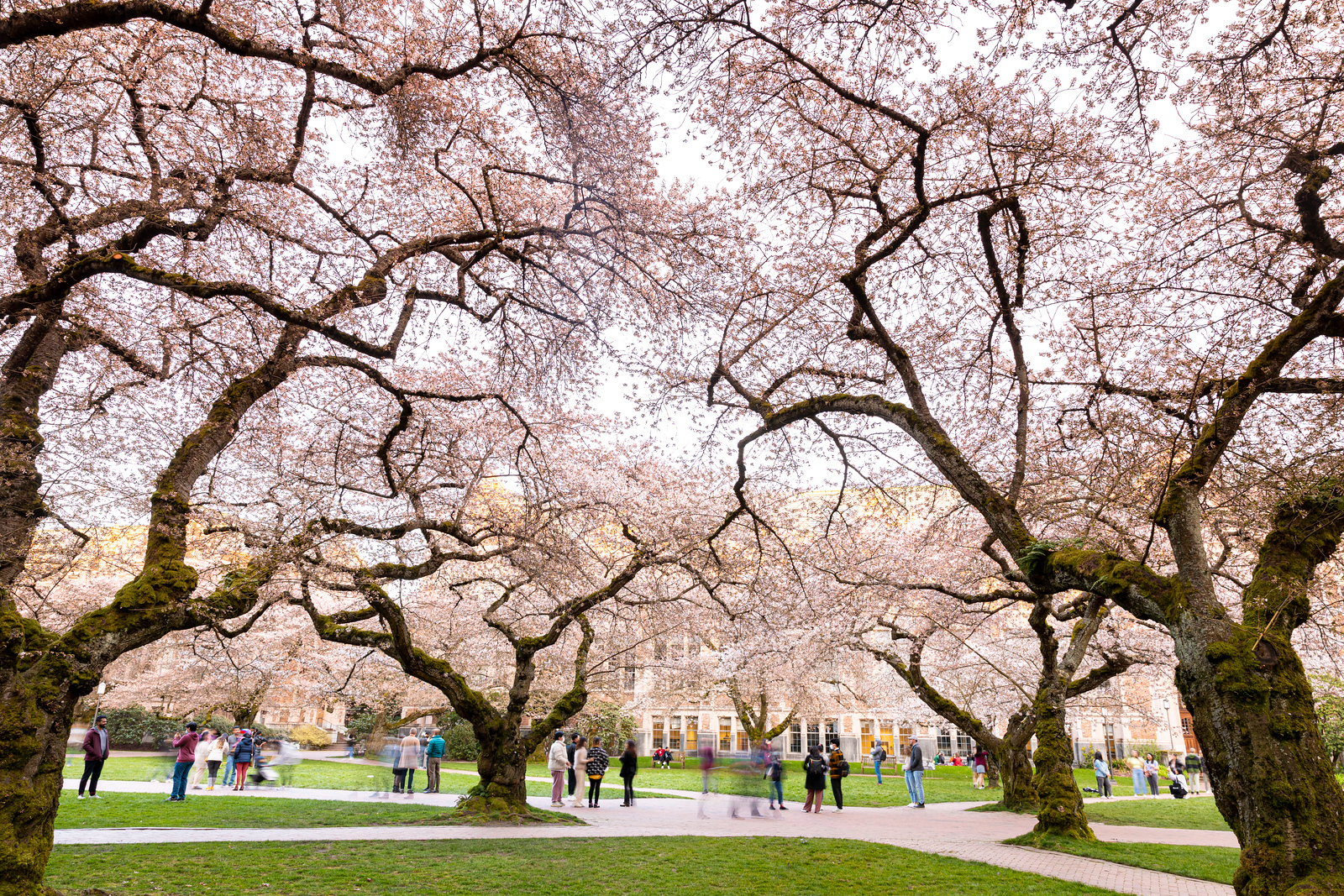
Sample grid item
Pudding candy canes muffin pastry jelly beans bonbon fruitcake.
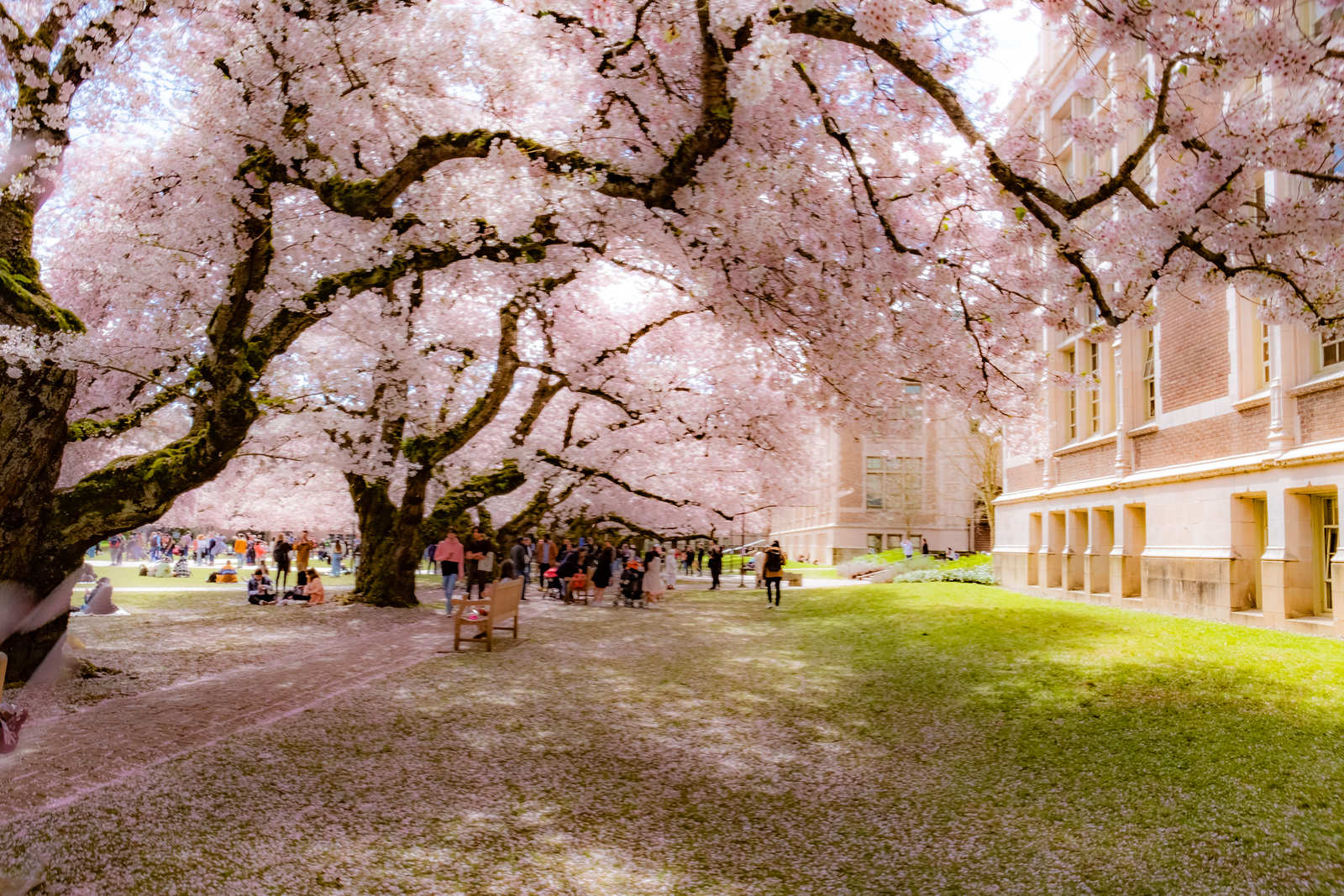
Sample grid item
Marzipan tootsie roll gummies dessert gummies soufflé danish.
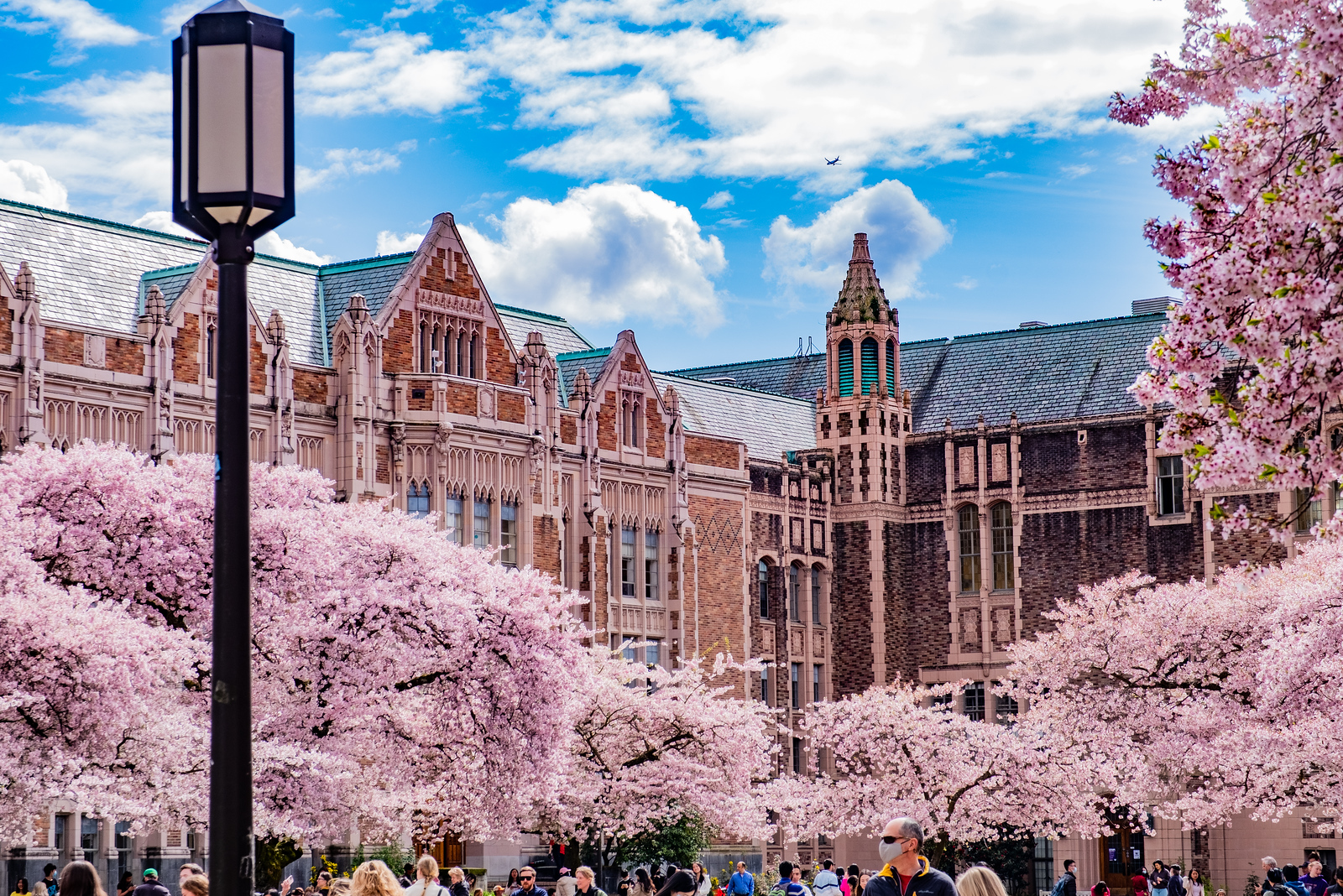
Sample grid item
Halvah toffee sweet oat cake cheesecake. Marzipan ice cream marzipan candy jujubes.
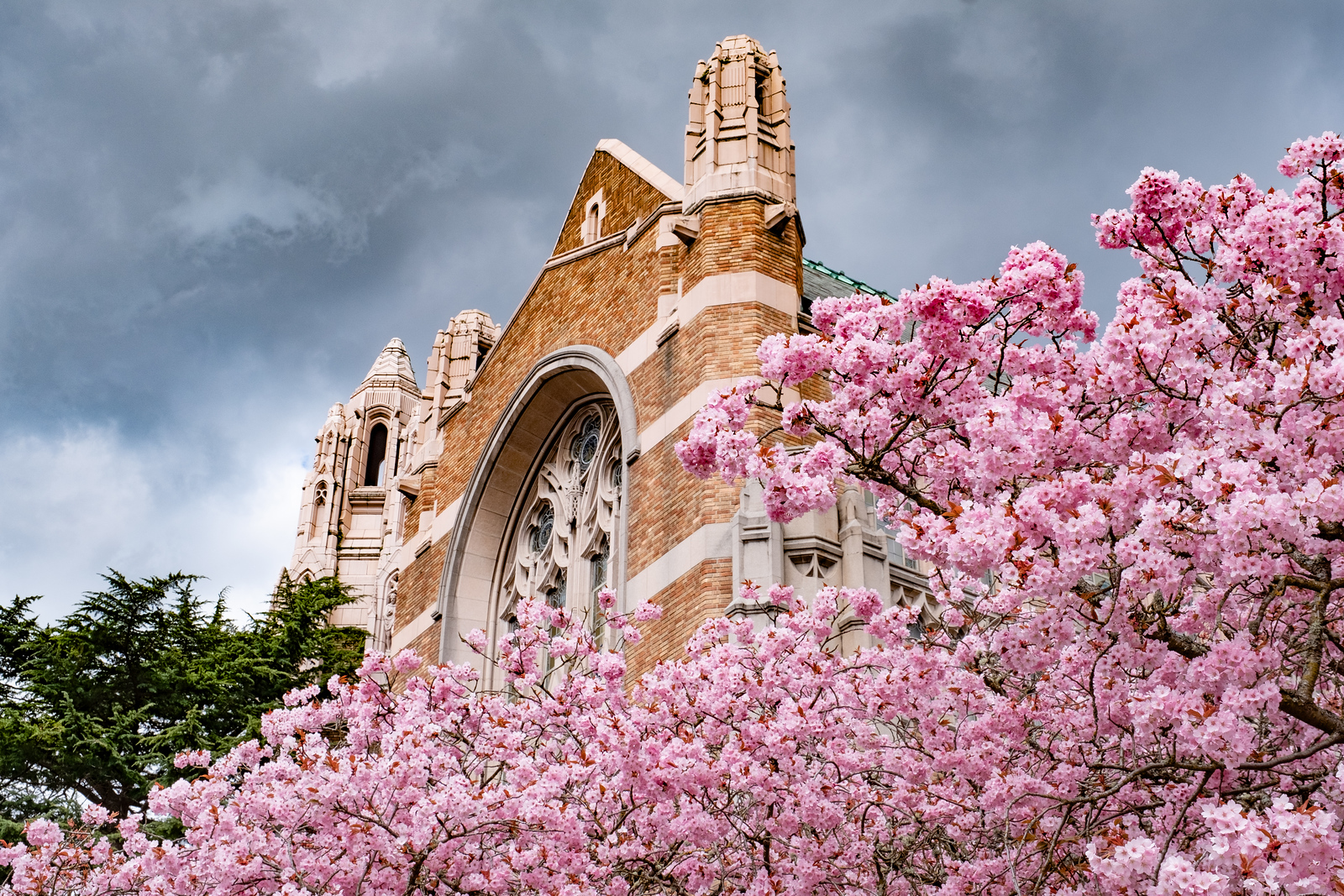
Sample grid item
Sugar plum tootsie roll fruitcake jelly-o carrot cake cake cake biscuit toffee.
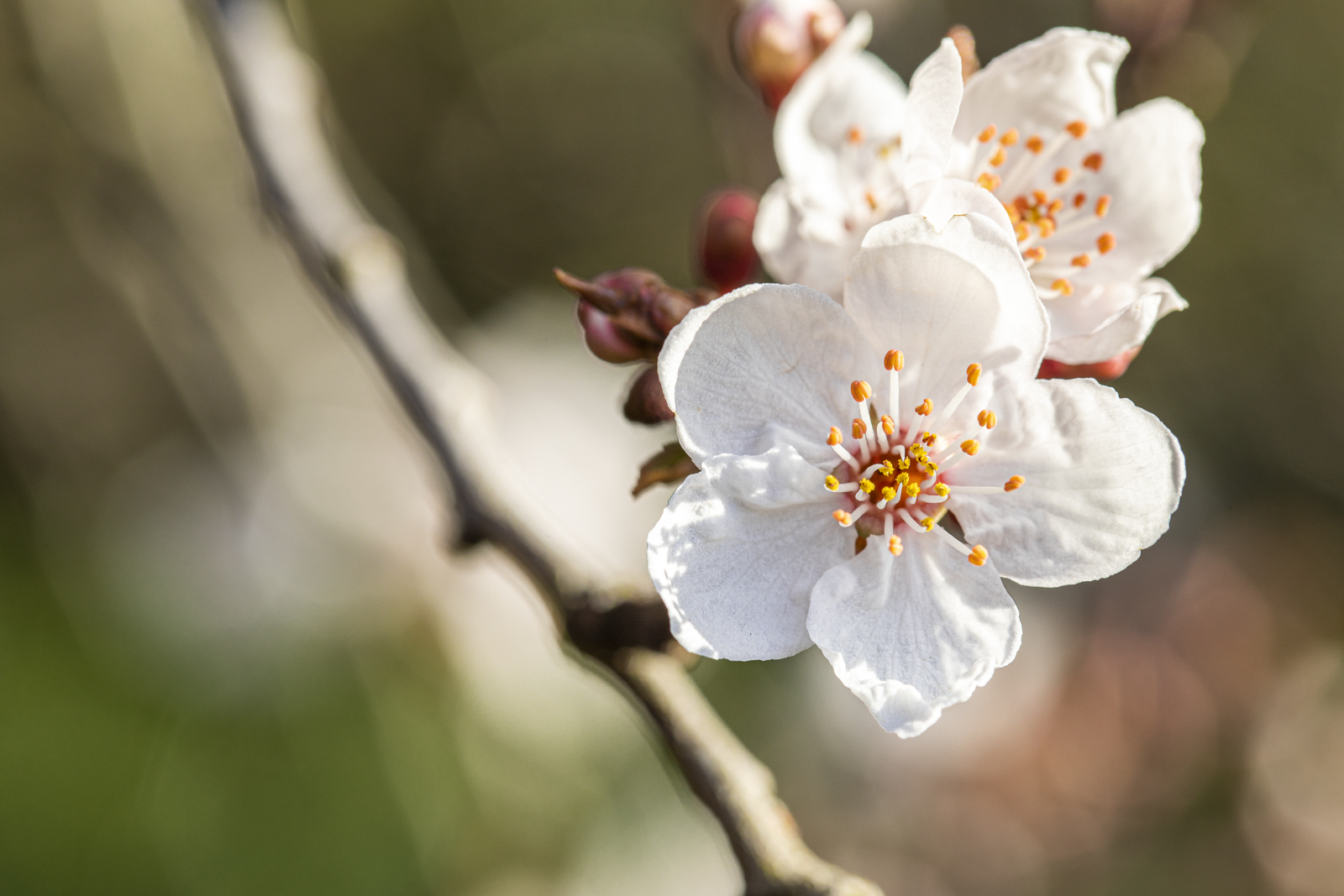
Sample grid item
Sweet roll topping jelly sweet carrot cake jujubes topping.
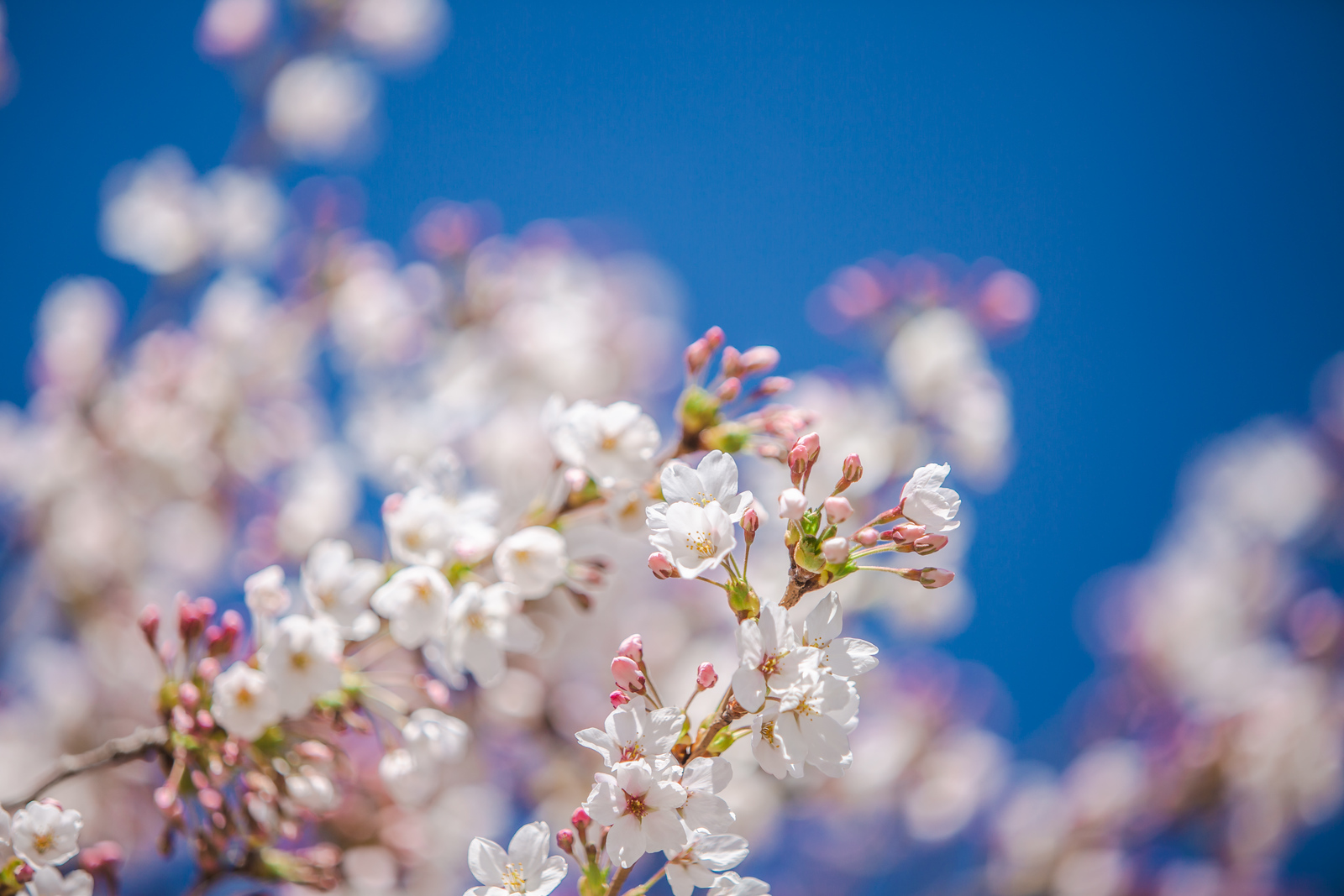
Sample grid item
Sweet roll topping jelly sweet carrot cake jujubes topping.
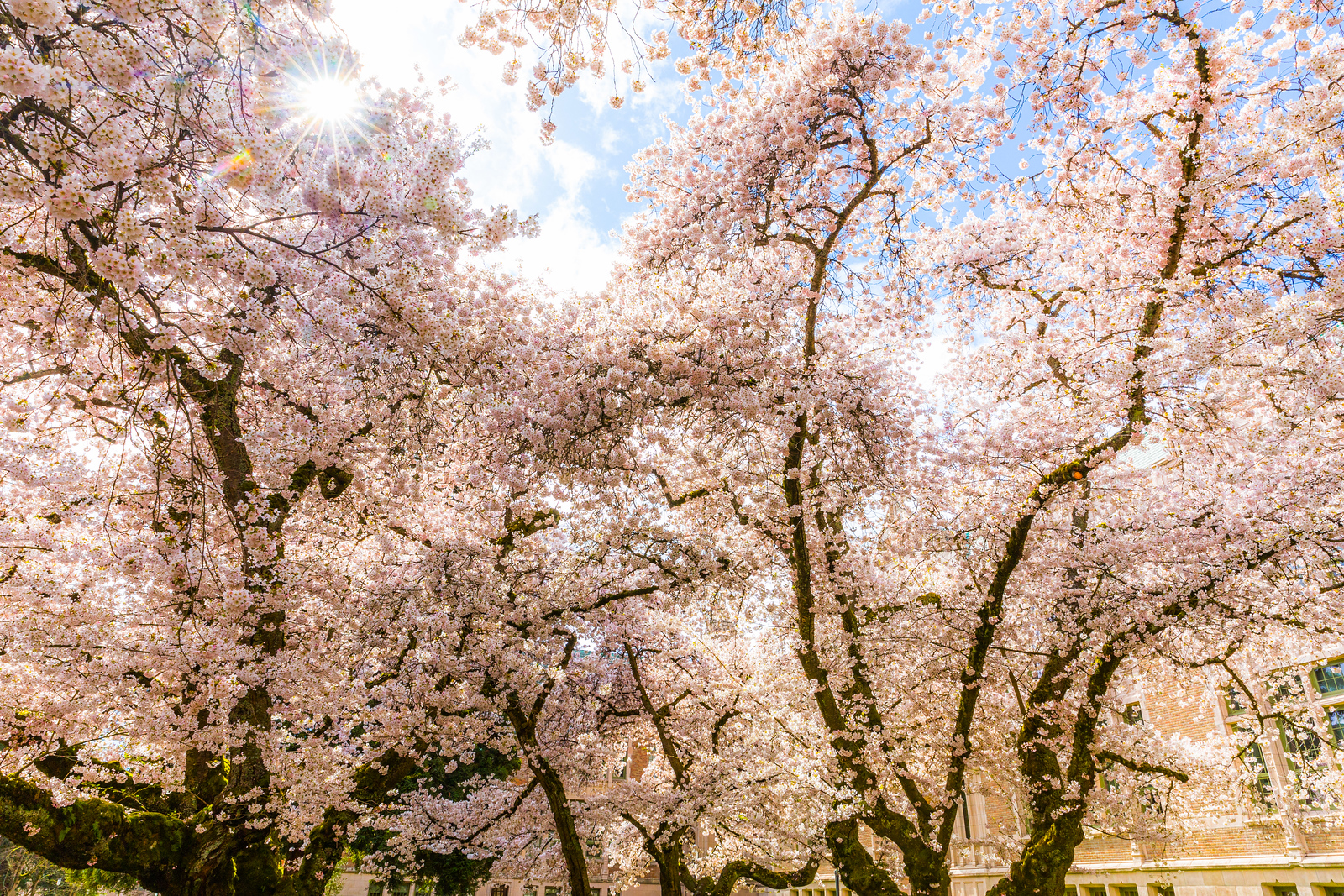
Sample grid item
Sesame snaps cupcake cookie bear claw tart pastry. Dragée chocolate cupcake macaroon powder fruitcake cookie liquorice toffee.
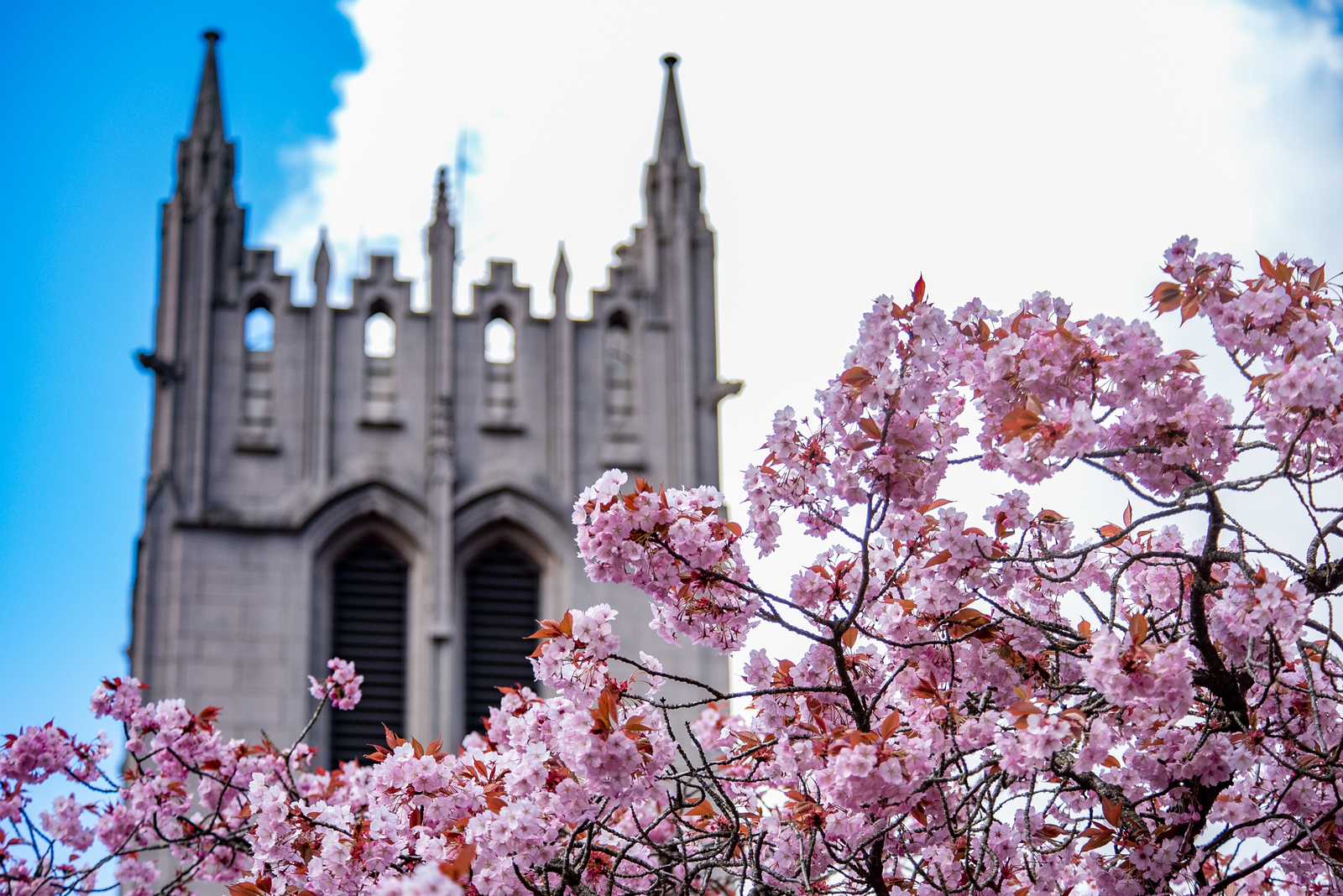
Sample grid item
Biscuit apple pie toffee pudding donut candy tart.
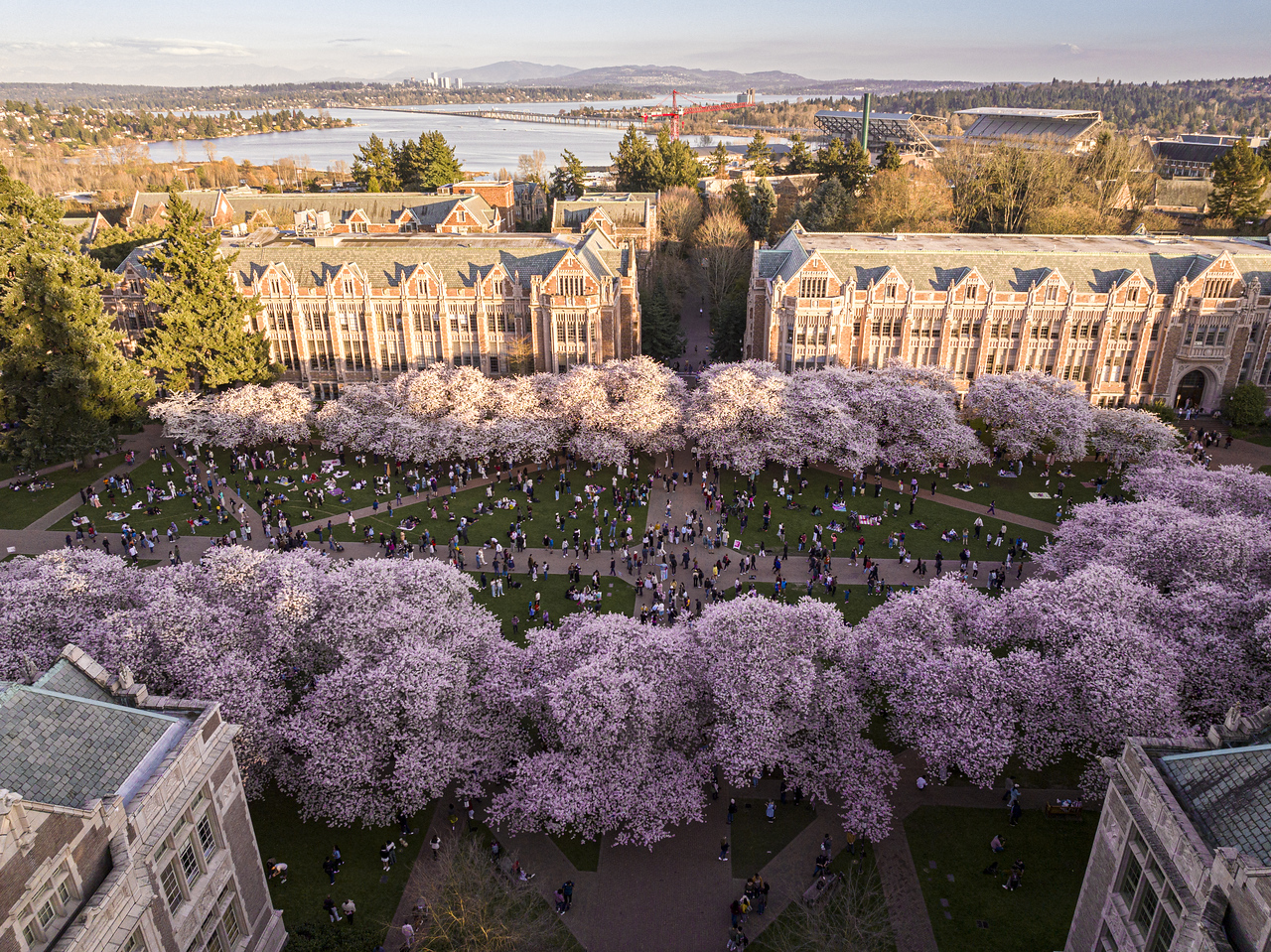
Sample grid item
Macaroon chupa chups muffin dessert jelly marzipan cheesecake.
Here is all the CSS that went into this example. Not much to it!
#grid-example {
.grid {
display: grid;
grid-template-columns: repeat(auto-fill, minmax(250px, 1fr));
gap: 1rem;
margin-bottom: 2rem;
.grid-item {
border: 1px solid #c1d640;
img {
width: 100%;
height: auto;
margin-bottom: 1rem;
}
h3, p {
padding-left: 1rem;
padding-right: 1rem;
}
}
}
}
Nested grid example
A nested grid is just using a grid inside of another grid. You aren't relying on the parent grid for the placement of child items. These grids are completely independent of each other, except that they happen to live inside an element that uses grid.
In this example, we are using the exact same markup from the previous example (plus adding a button!) but you can see we have a completely different layout. Almost all courtesy of grid
.
In your Dev Tools, under Elements > Layout, check the boxes for this .grid
and any of the .grid-item
elements to see how the grid are structured with grid lines. Play with the grid layout options to see what looks best.
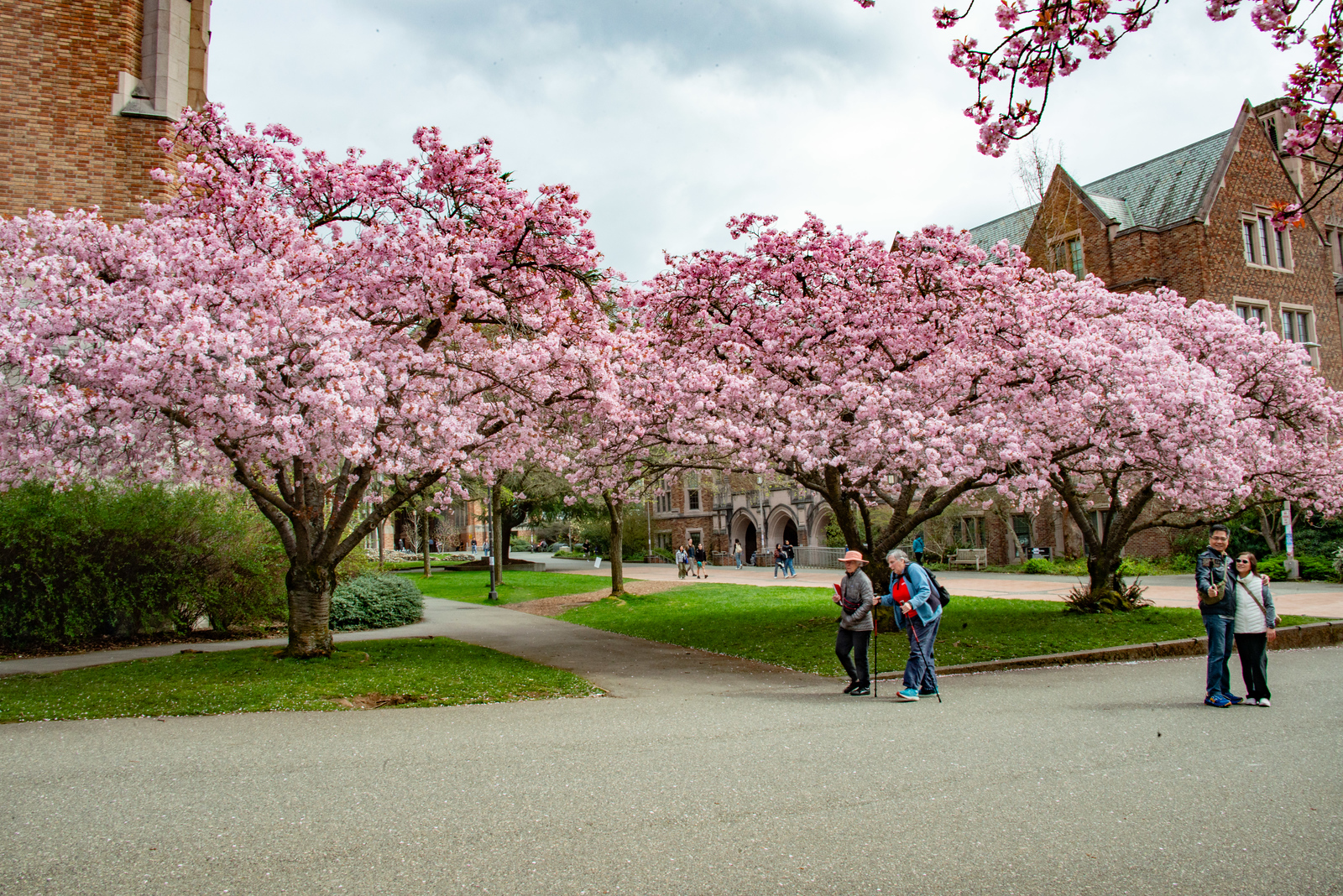
Sample grid item
Cupcake ipsum dolor sit amet. Liquorice bear claw tiramisu sweet shortbread.
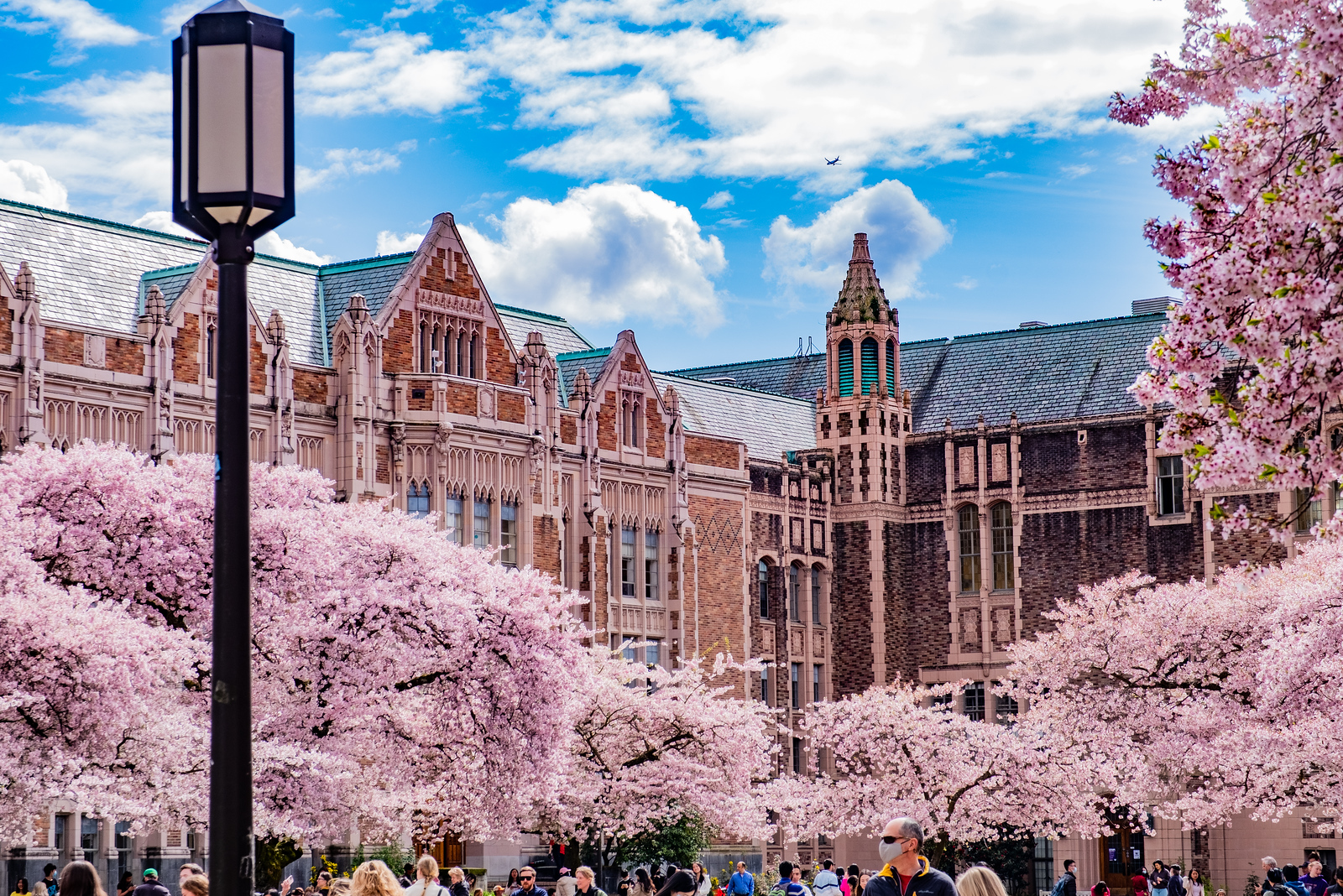
Sample grid item
Halvah toffee sweet oat cake cheesecake. Marzipan ice cream marzipan candy jujubes.
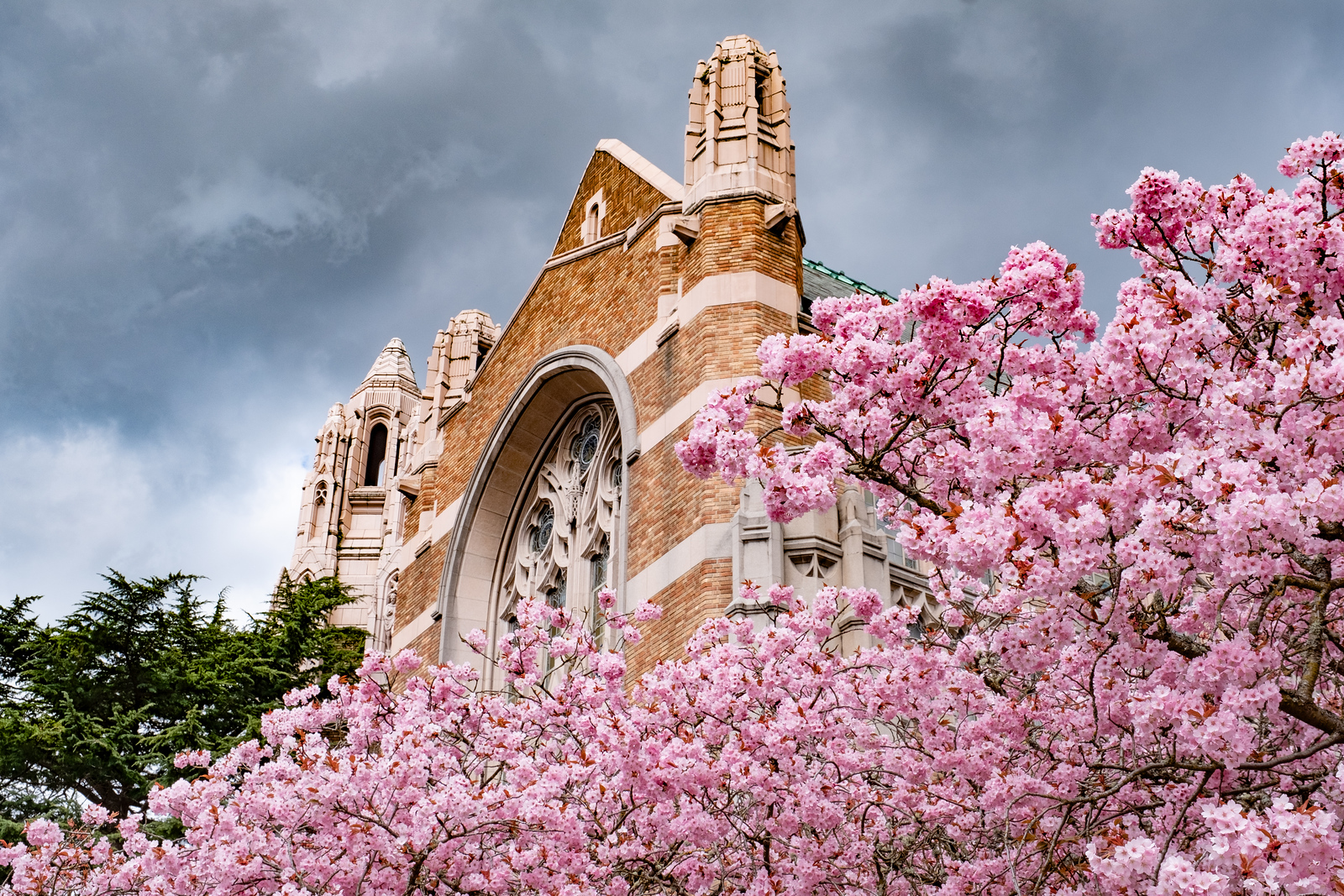
Sample grid item
Sugar plum tootsie roll fruitcake jelly-o carrot cake cake cake biscuit toffee.
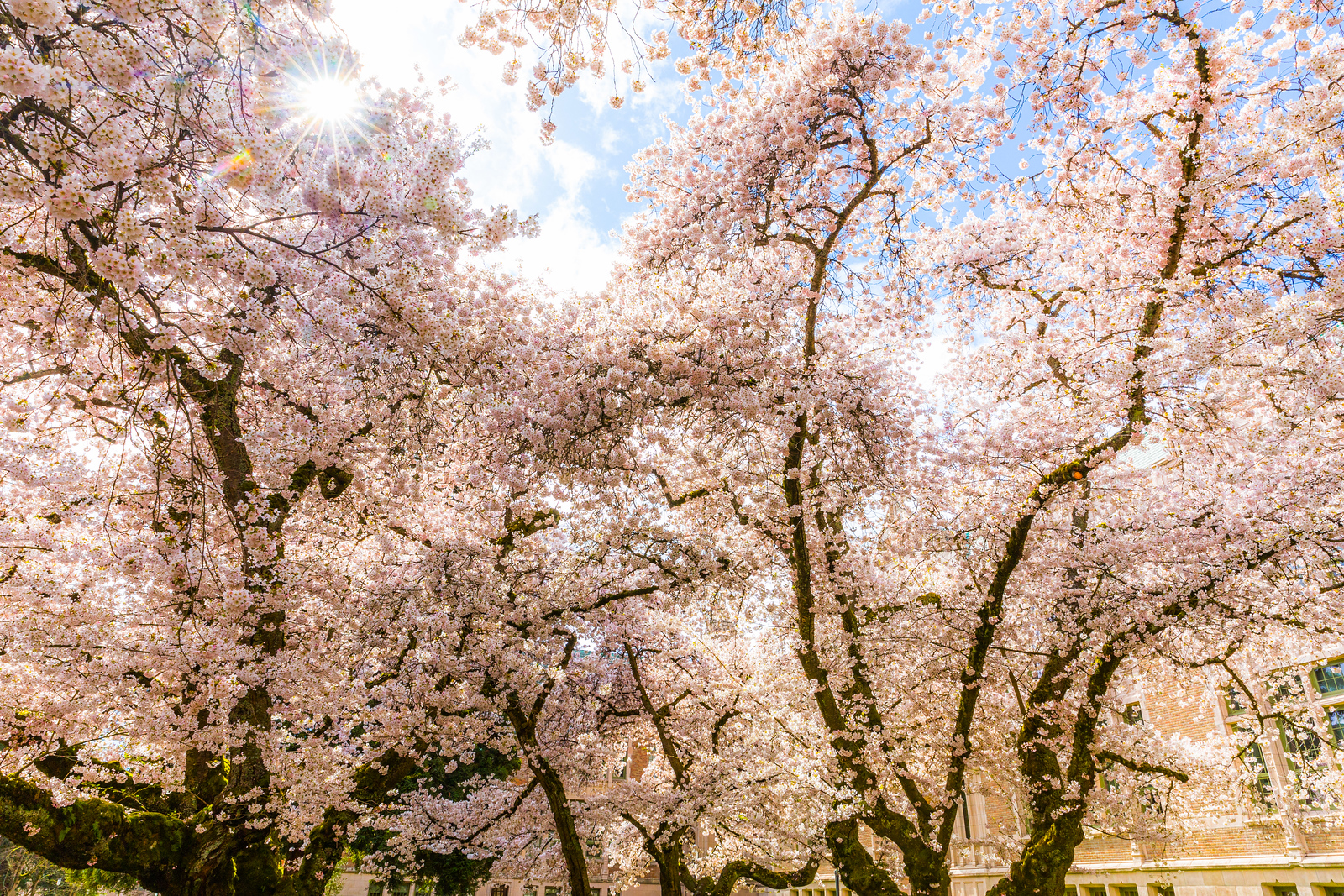
Sample grid item
Sesame snaps cupcake cookie bear claw tart pastry. Dragée chocolate cupcake macaroon powder fruitcake cookie liquorice toffee.
Our CSS:
#nested-grid-example {
.grid {
display: grid;
grid-template-columns: repeat(auto-fill, minmax(500px, 1fr));
gap: 1rem;
margin-bottom: 2rem;
.grid-item {
border: 1px solid #33b1a8;
display: grid;
grid-template-columns: 2fr 1fr;
img {
width: 100%;
height: 100%;
object-position: center;
object-fit: cover;
grid-row-start: 1;
grid-row-end: 4;
}
h3, p {
padding-left: 1rem;
padding-right: 1rem;
}
}
}
}
Subgrid example — generic
Now, say you want to your child grid items to use the parent grid for placement. That's where subgrid
comes into play!
Because subgrid is all about placement, we'll need to use a new example. Open up your Dev Tools and peek around to see what's going on with this grid.
Sample grid item
Cupcake ipsum dolor sit amet. Liquorice bear claw tiramisu sweet shortbread.
Note: this example was lifted from MDN.
And here is our CSS:
#subgrid-example-1 {
.grid {
display: grid;
grid-template-columns: repeat(12, 1fr);
grid-template-rows: repeat(4, minmax(100px, auto));
gap: 1rem;
margin-bottom: 2rem;
background-color: #ddd;
border: 1px solid #333;
.grid-item {
border: 1px solid #eb4B99;
background-color: pink;
display: grid;
grid-column: 2 / 7;
grid-row: 2 / 4;
grid-template-columns: subgrid;
grid-template-rows: repeat(3, 80px);
.subitem {
grid-column: 3 / 6;
grid-row: 1 / 3;
border: 1px solid #33b1a8;
background-color: lightblue;
}
}
}
}
So this is great and all but I'm guessing you want a practical example?
Subgrid example — specific use case
An example of where you might use subgrid is to create a uniform card layout, with automatically equal height elements in each row based on the content.
In this example:
- The "Read more" link is stuck to the bottom of the card in the same location for every card, independent of the height of the content above it.
- The heading sections are all the same height, based on the tallest one in the row.
- The content sections are equal height based on the longest copy block.
Let's first look at the example with some background colors applied so it's easier to see the different elements.
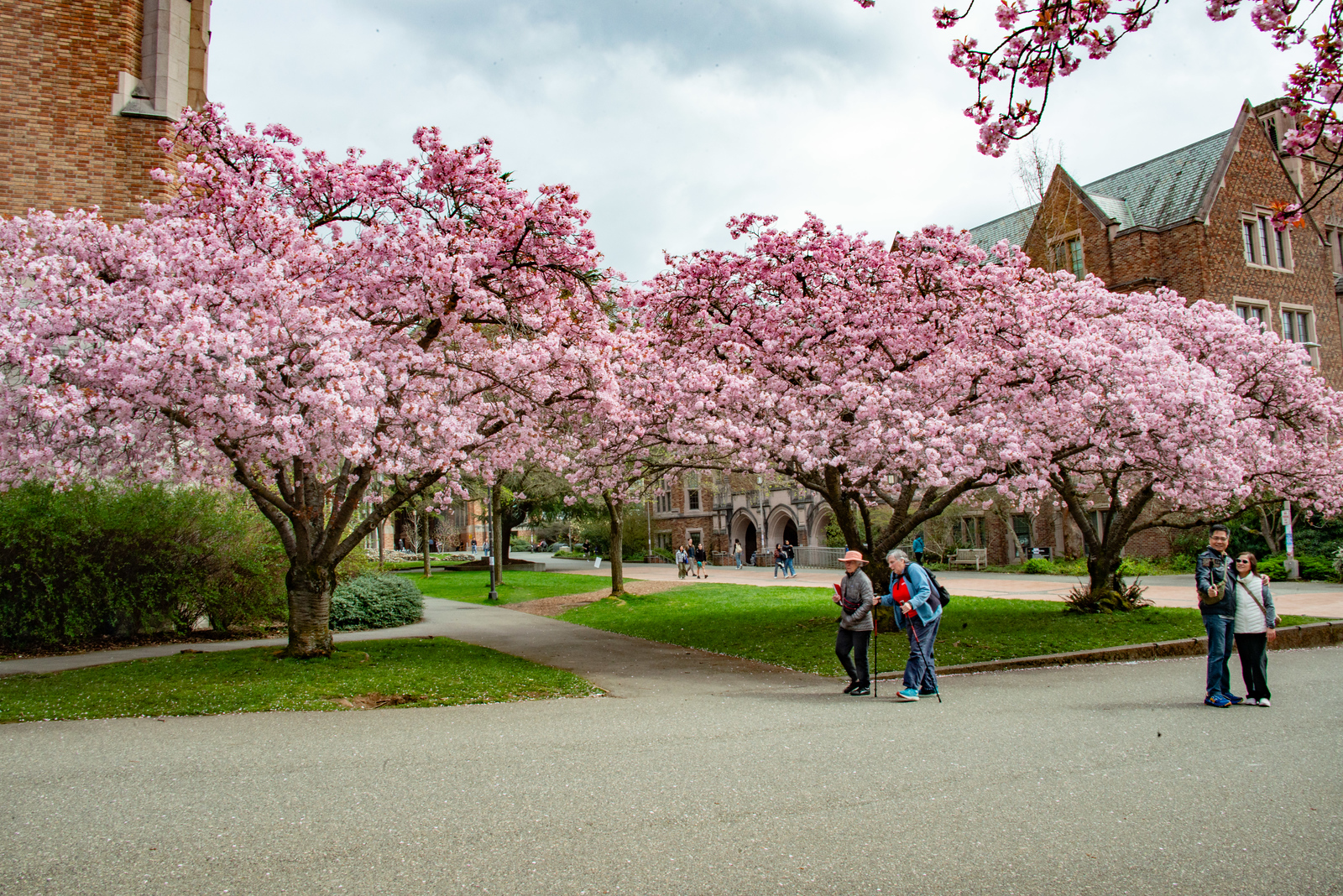
Sample grid item
Cupcake ipsum dolor sit amet. Liquorice bear claw tiramisu sweet shortbread.
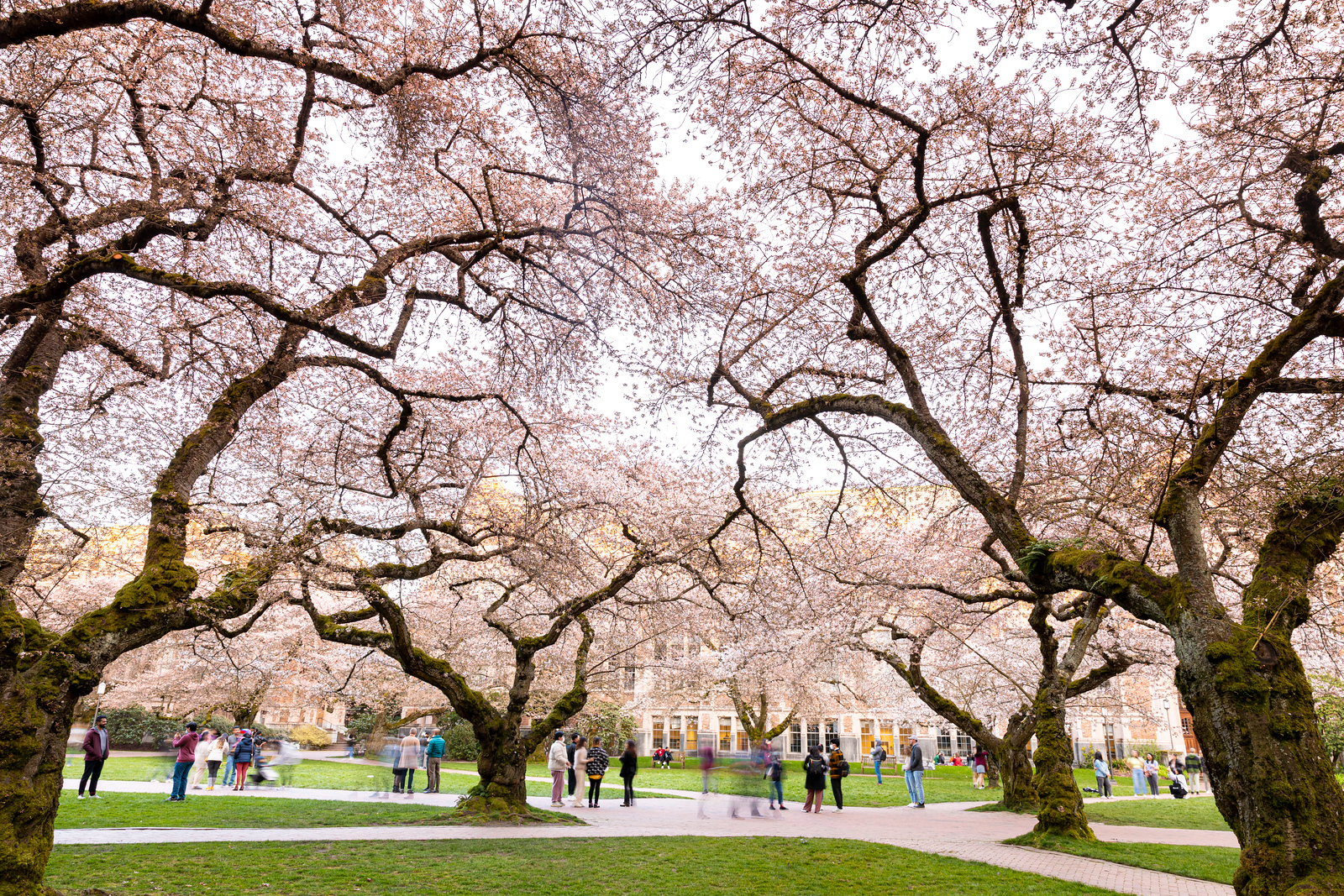
Sample grid item with a longer title
Pudding candy canes muffin pastry jelly beans bonbon fruitcake. Marzipan tootsie roll gummies dessert gummies soufflé danish.
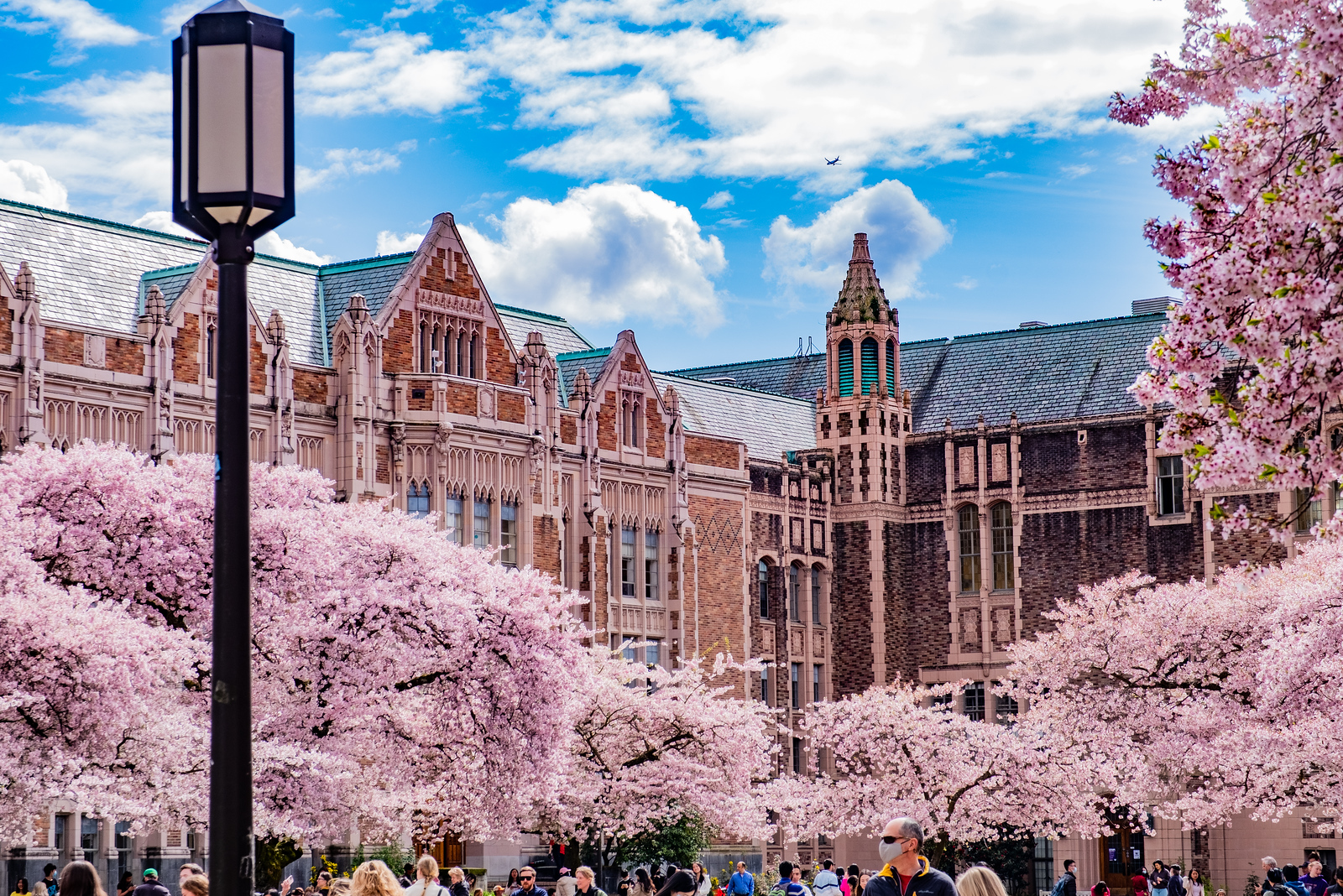
Short title
Halvah toffee sweet oat cake cheesecake. Marzipan ice cream marzipan candy jujubes.
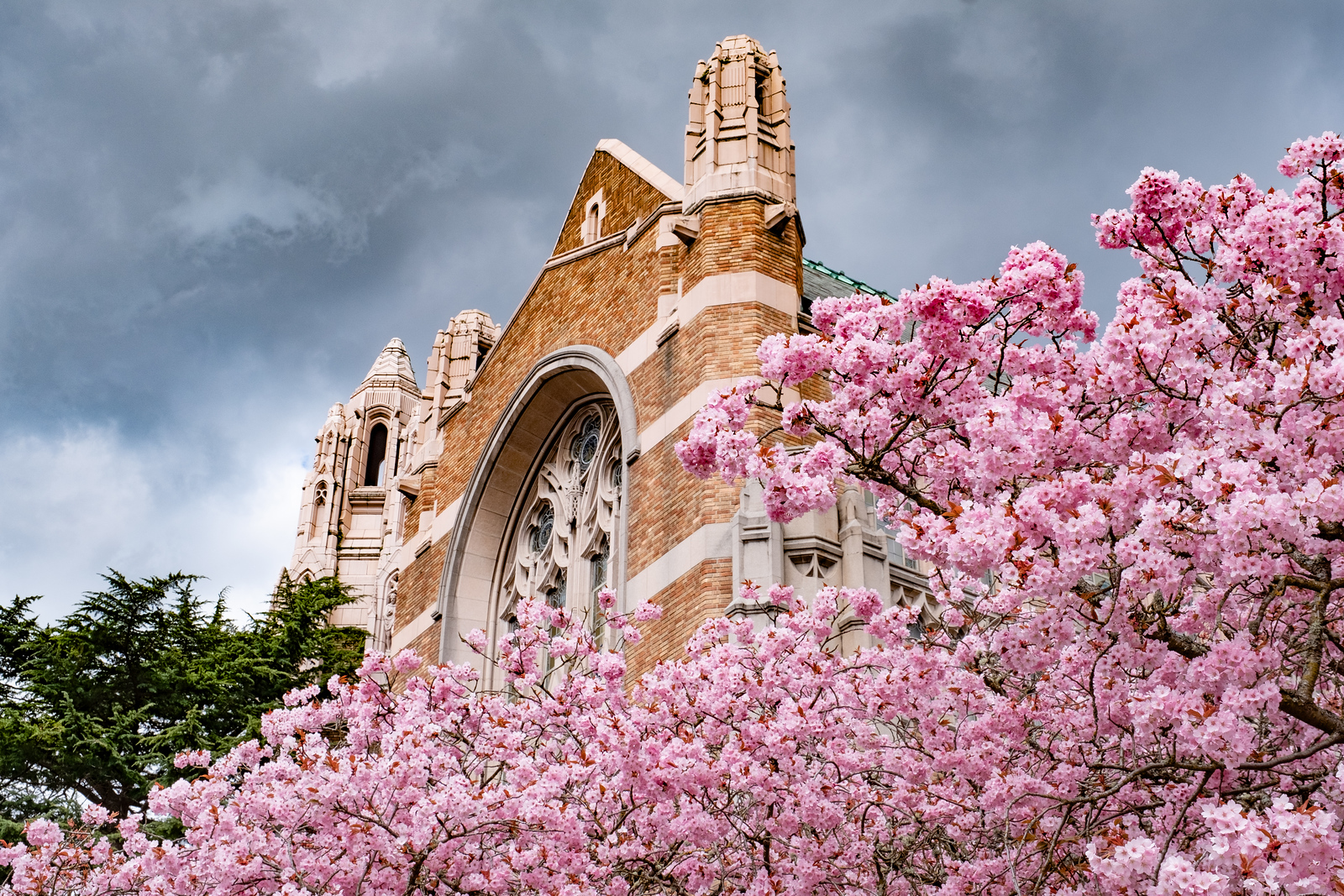
Sample grid item
Sugar plum tootsie roll fruitcake jelly-o carrot cake cake cake biscuit toffee.
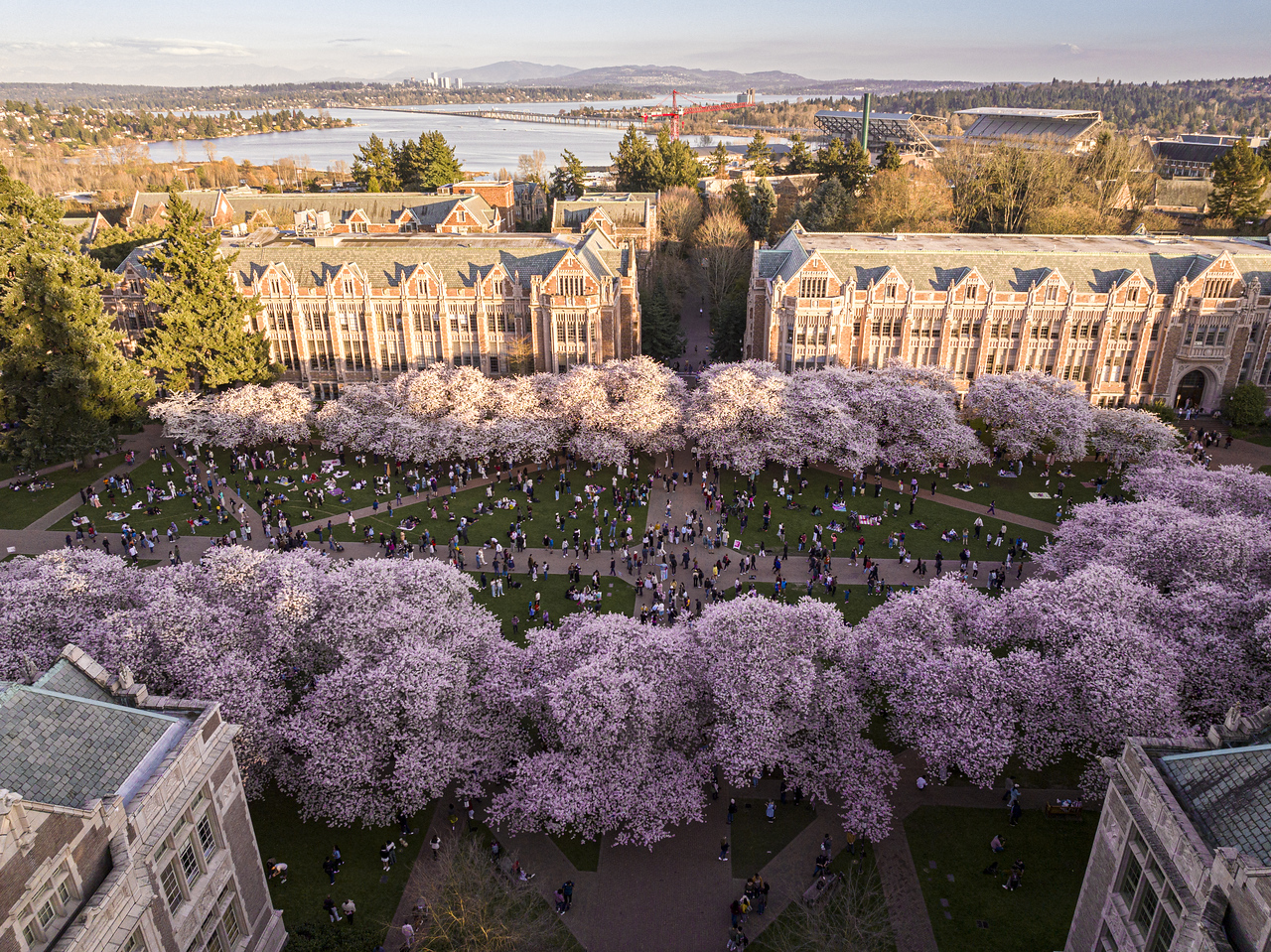
Sample grid item
Macaroon chupa chups muffin dessert jelly marzipan tiramisu apple pie toffee cheesecake.
So, how did we accomplish this? Let's take a look at the CSS and walk through it together:
#subgrid-example-2 {
.grid {
display: grid;
grid-template-columns: repeat(auto-fill, minmax(200px, 1fr));
gap: 1rem;
margin-bottom: 2rem;
.grid-item {
display: grid;
grid-template-rows: subgrid;
grid-row: span 4;
gap: 0;
border: 1px solid var(--wp--preset--color--spirit-purple);
img {
width: 100%;
height: 200px;
object-position: center;
object-fit: cover;
}
h3, p {
padding-left: 1rem;
padding-right: 1rem;
margin: 0;
}
h3 {
background-color: lightskyblue;
}
p {
background-color: lightpink;
}
p:has(a) {
background-color: lightgreen;
a { color: darkblue; }
}
}
}
}
Now we can see the same example without the background colors. Note: the only CSS change here is removing the backgrounds!
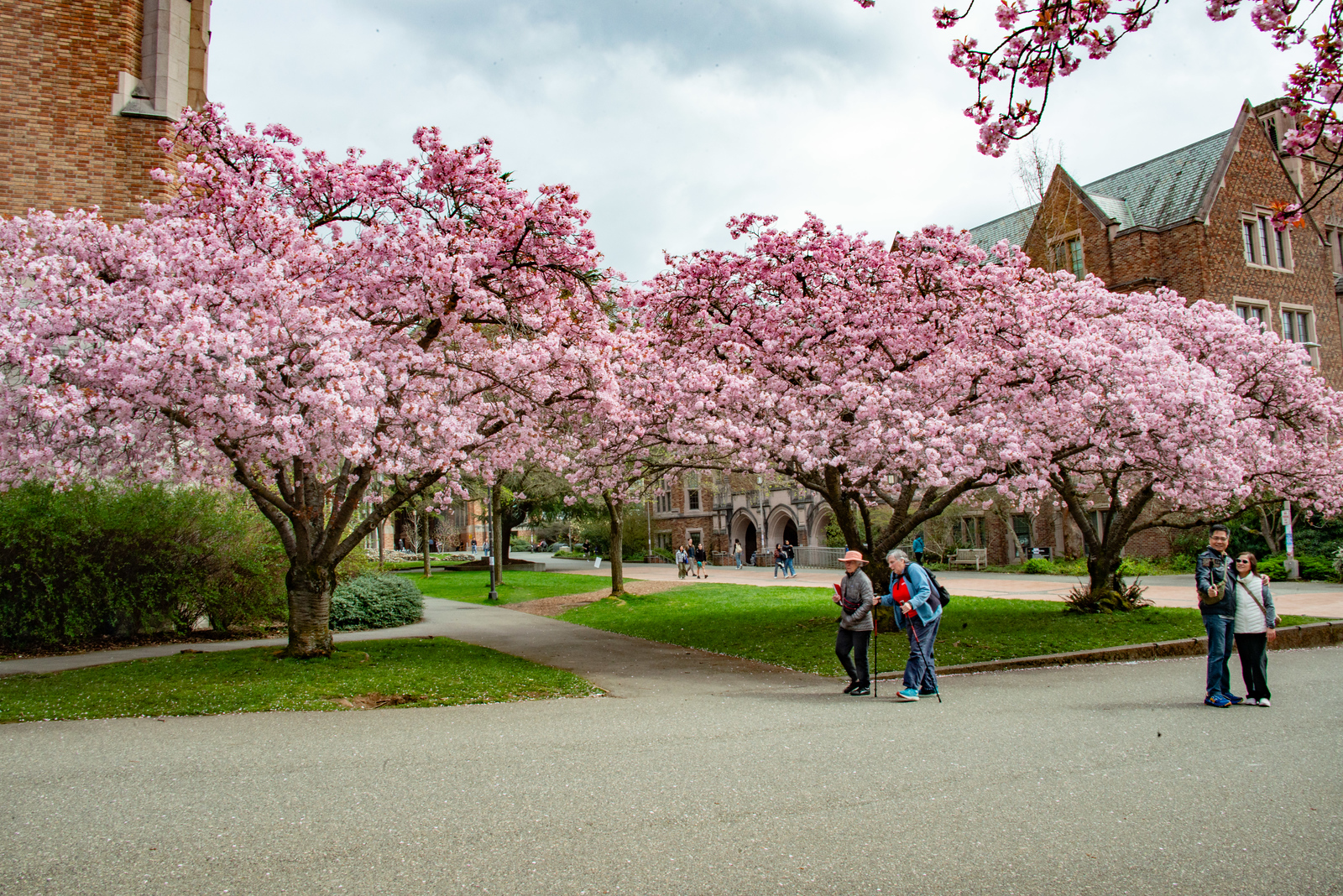
Sample grid item
Cupcake ipsum dolor sit amet. Liquorice bear claw tiramisu sweet shortbread.
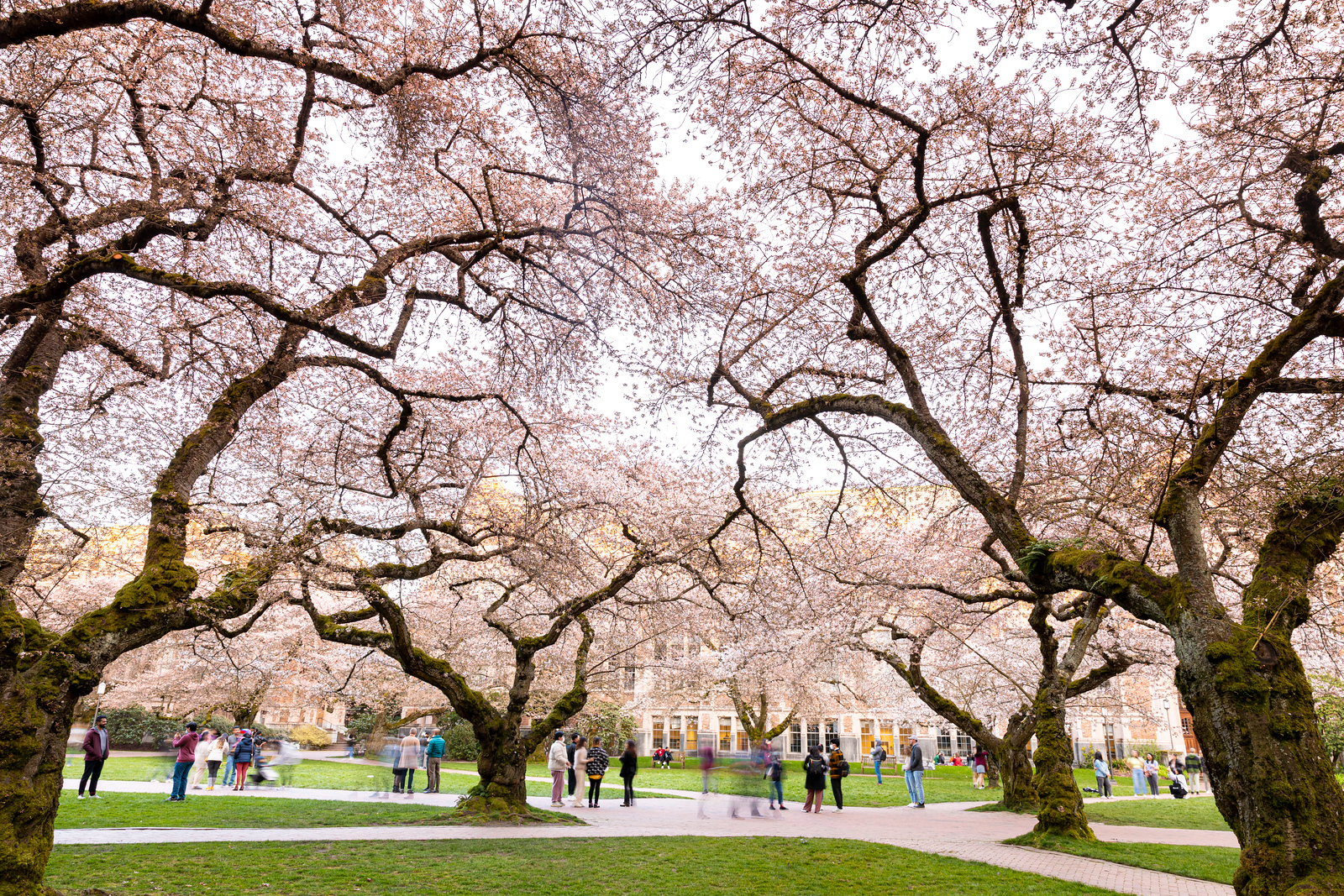
Sample grid item with a longer title
Pudding candy canes muffin pastry jelly beans bonbon fruitcake. Marzipan tootsie roll gummies dessert gummies soufflé danish.
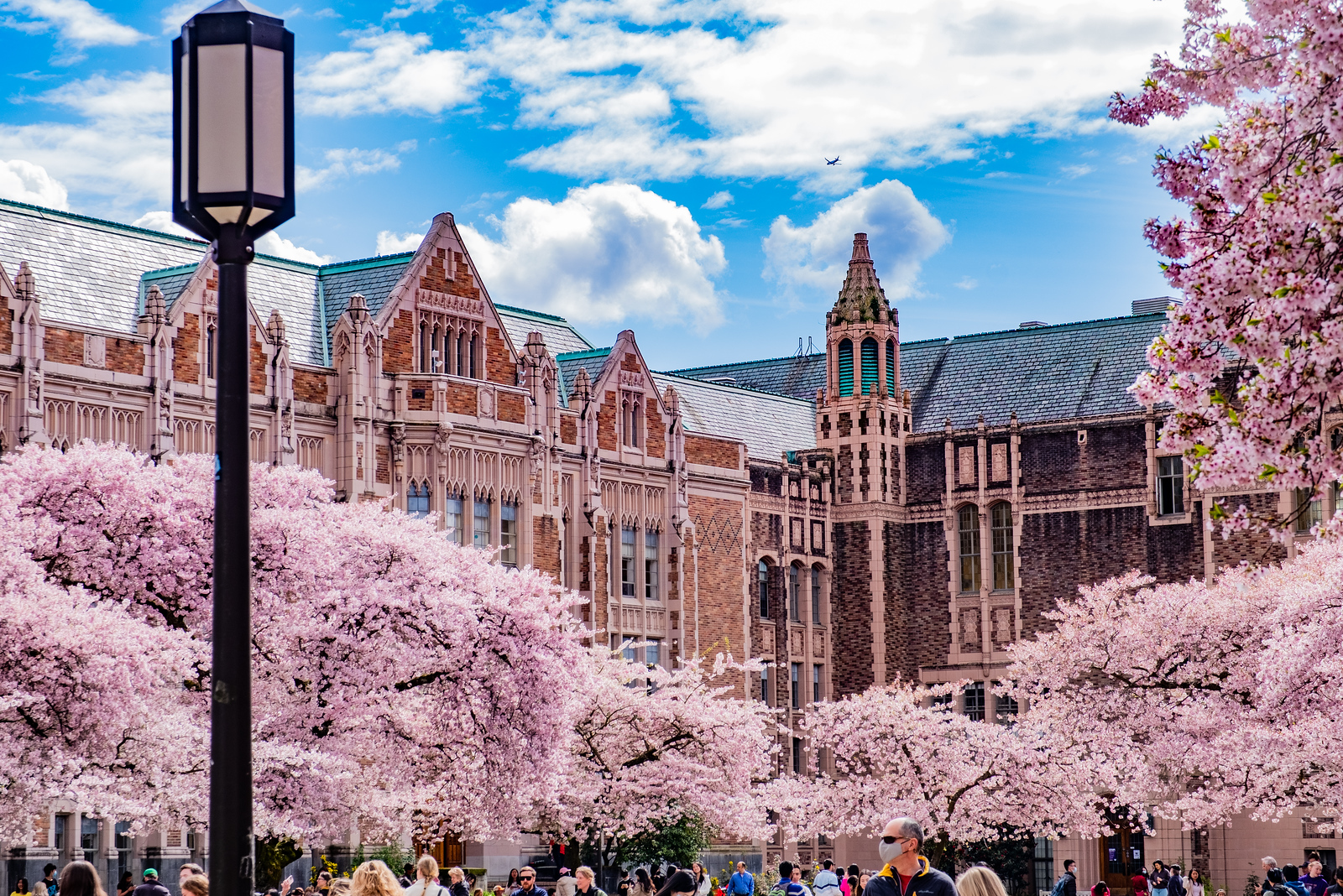
Short title
Halvah toffee sweet oat cake cheesecake. Marzipan ice cream marzipan candy jujubes.
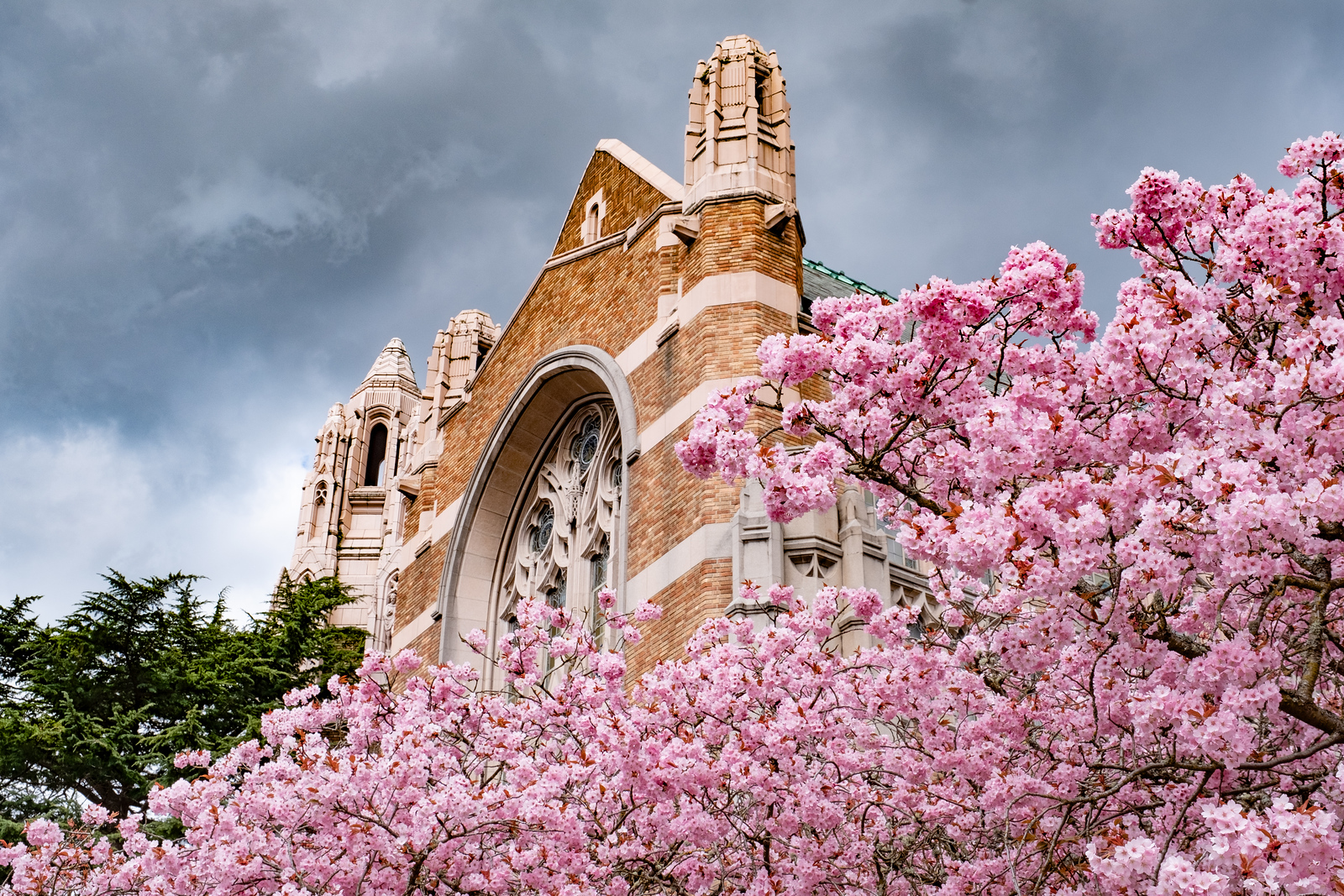
Sample grid item
Sugar plum tootsie roll fruitcake jelly-o carrot cake cake cake biscuit toffee.
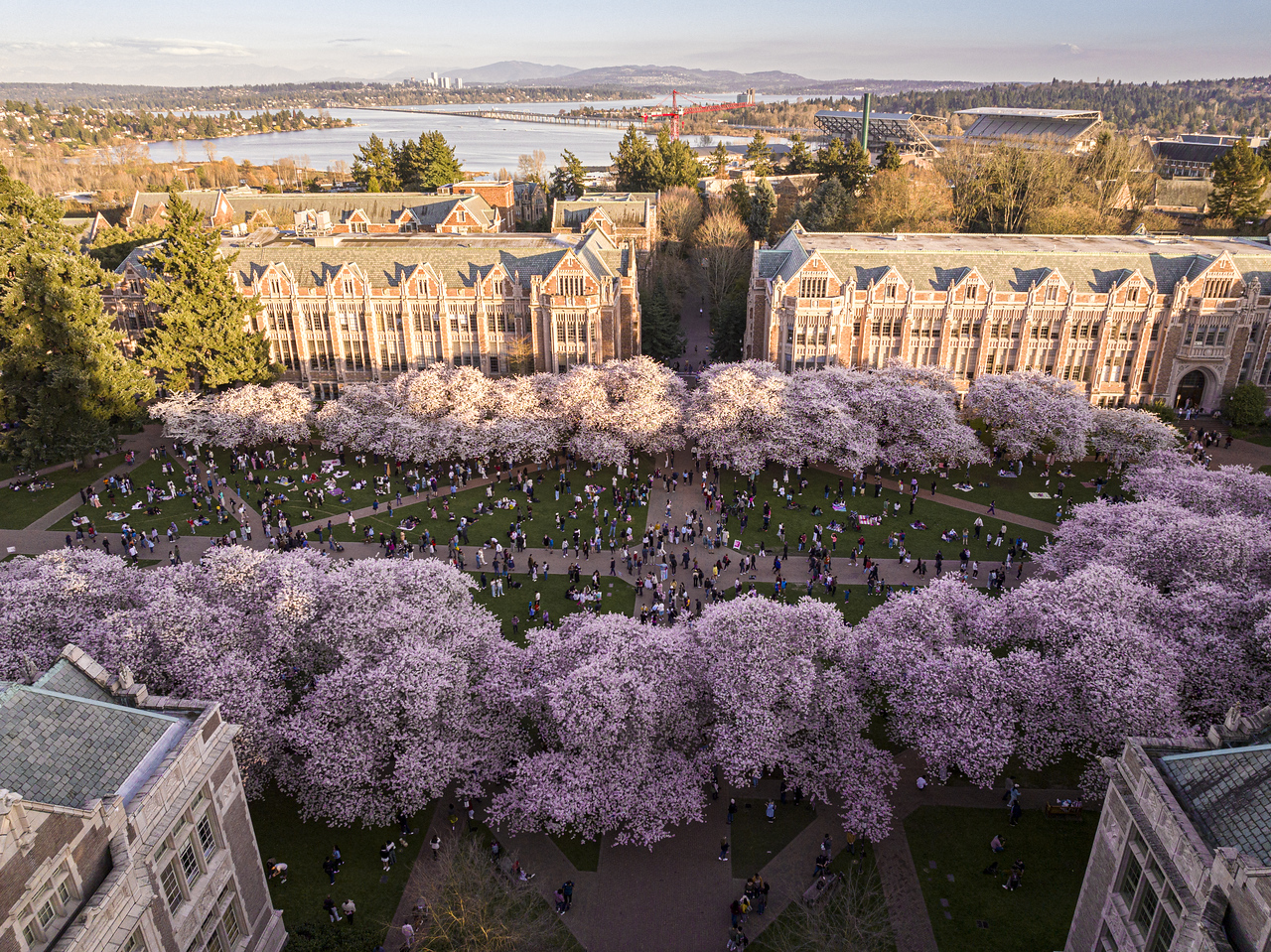
Sample grid item
Macaroon chupa chups muffin dessert jelly marzipan tiramisu apple pie toffee cheesecake.