Here are two articles to read:
- Cross-domain communications with JSONP, Part 1: Combine JSONP and jQuery to quickly build powerful mashups
- Cross-domain communications with JSONP, Part 2: Building mashups with JSONP, jQuery, and Yahoo! Query Language
Mashup - Content from various web sources presented ensemble
JSONP - JavaScript Simple Object Notation with Padding.
jQuery - JavaScript library
YQL - Yahoo! Query Language
The same domain restriction
JavaScript uses a "same origin policy" that permits web pages that share a common domain to interact, but restricts interaction among pages from different domains.
This web page, however, is a mashup web page from the domain: "projects.ischool.washington.edu" that contains content from several pages from other domains. The content from those pages is injected into this page by jQuery scripts that make JSONP calls to YQL. Upon receipt of a payload, jQuery patches the content into this web page. In effect, JSONP is a cross-domain communication technique that bypasses the same-origin policy limitation.
Note that the content in the orange box below originates in the domain: "faculty.washington.edu" and is the src attribute of the red square on my home page.
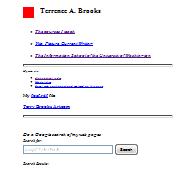
Note that the content in the orange box below originates in the domain: "en.wikipedia.org" and is the color of an Alaskan Malamute dog. This content is located inside a paragraph element that is inside a table data element located in the InfoBox feature on the righhand-side of the Wikipedia web page: "Alaskan Malamute".
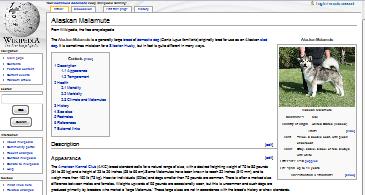
YQL - Yahoo! Query Language
The YQL Web Service acts as a facilitator by reaching out for web content and then re-packaging it as JSONP that can be injected into a web page. "Cross-domain communications with JSONP" visualizes YQL this way:"
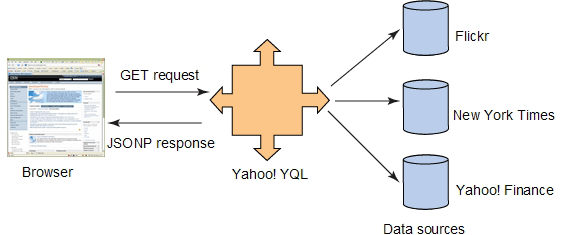
You use Yahoo! Query Language to target a web source and, in combination with an XPath, the exact content from the web source you desire. The YQL console permits you to test your YQL search statement and anticipate its exact JSONP payload. By using XPath in the YQL search statement, you can determine the precise content that you want from the web page.
XPather is just terrific for creating an XPath for the desired content. Go to your target page and click on the stuff you want and then mouse right click to get the XPath to the stuff you want.
For example, show below is the YQL console executing the following search statement
select * from html where url = "http://en.wikipedia.org/wiki/Alaskan_Malamute" and xpath = "//table[@class='collapsible']/tr[3]/td/p"
This search statement requests the Alaskan Malamute web page from Wikipedia and then fires an XPath into this web page to target the contents of a paragraph element. It produces this JSON object
{
"query": {
"count": "1",
"created": "2009-06-29T09:52:56Z",
"lang": "en-US",
"updated": "2009-06-29T09:52:56Z",
"uri": "http://query.yahooapis.com/v1/yql?q=select+*+from+html+where+url+
%3D+%22http%3A%2F%2Fen.wikipedia.org%2Fwiki%2FAlaskan_Malamute%22+and+xpath+
%3D+%22%2F%2Ftable%5B%40class%3D%27collapsible%27%5D%2Ftr%5B3%5D%2Ftd%2Fp%22",
"diagnostics": {
"publiclyCallable": "true",
"url": {
"execution-time": "30",
"content": "http://en.wikipedia.org/wiki/Alaskan_Malamute"
},
"user-time": "36",
"service-time": "30",
"build-version": "1949"
},
"results": {
"p": "Gray, sable, black, or red, always with white"
}
}
}
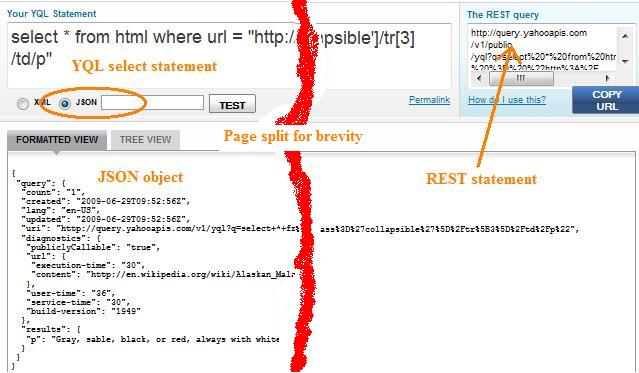
When your YQL search statement is correct and you are receiving the desired payload, you can use the REST statement produced by the YQL console in your web page jQuery code.
Web page jQuery
The same origin policy doesn't prevent the insertion of dynamic script elements into a web page. It is possible to insert dynamic function calls from different domains: JSONP is JSON data wrapped in a web page function call - JSONP: JSON with Padding. YQL serves the purpose of communicating with some web source and then structuring the web source as a JSON object. Web page jQuery originates the YQL request and provides the callback function for the JSONP payload.
// Alaskan Malamute from DBPedia $.getJSON( "http://query.yahooapis.com/[ the YQL search statement here ] &format=json&callback=?", function(json){ $('#dog').text(json.query.results.td.p); // Patching payload into page element ID = "dog" });