Greasemonkey is an extension of Firefox. You can write a JavaScript in the form of a Greasemonkey "user script" that your Firefox browser applies to the DOM just before the HTML is painted on the browser screen. This means that you have everything available to you: the HTML code, CSS style code and any JavaScripts attached to the webpage.
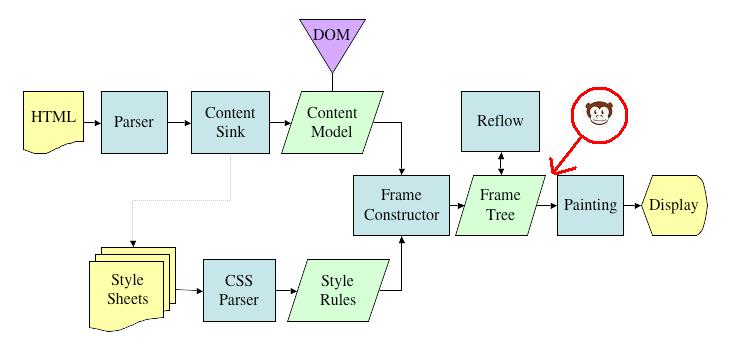
Download Greasemonkey [Use your Firefox browser]
A Greasemonkey scripting template
A Greasemonkey tutorial
A scripter's comparison of XPath and DOM methods
Firefox extensions useful with Greasemonkey
XPath - XML Path Language
This is a language for selecting nodes from an XML document. The XPath language is based on a tree representation of the XML document, and provides the ability to navigate around the tree, selecting nodes by a variety of criteria.
<?xml version="1.0" encoding="ISO-8859-1"?> <bookstore> <book> <title lang="eng">Harry Potter</title> <price>29.99</price> </book> <book> <title lang="eng">Learning XML</title> <price>39.95</price> </book> </bookstore>
Expression | Description |
---|---|
/ | Selects from the root node |
// | Selects nodes in the document from the current node that match the selection no matter where they are |
. | Selects the current node |
.. | Selects the parent of the current node |
@ | Selects attributes |
Path Expression | Result |
---|---|
/bookstore | Selects the root element bookstore Note: If the path starts with a slash ( / ) it always represents an absolute path to an element! |
bookstore/book | Selects all book elements that are children of bookstore |
//book | Selects all book elements no matter where they are in the document |
bookstore//book | Selects all book elements that are descendant of the bookstore element, no matter where they are under the bookstore element |
//@lang | Selects all attributes that are named lang |
While our course is not focused on extensible technologies, you may be interested in seeing examples of how XPath can be used in targeting information nodes in XML documents. Here's a web page about XPath that I used in our course Winter 2006.
Here's a page where you can play with XPath queries.
Replace the picture of the "greasemonkey" on this page with a picture of "Dubs", the UW mascot.
University of Washington mascot Dubs has a webpage with a handsome photo. Point your browser at Dubs to view the noble canine. Note that the domain of this photo is "www.gohuskies.com", while the domain of the webpage that you're reading at this moment is "projects.washington.ischool.edu".
The following Greasemonkey script fires on this webpage. It locates the <img> tag of the greasemonkey. It reaches out to Dub's page and downloads its HTML. It finds the name of Dub's picture. It asks the gohuskies.com server for the picture. It pastes this address into this webpage. Then your browser repaints this webpage on your browser screen. Presto! gummo! Dubs is on this page instead of the greasemonkey.
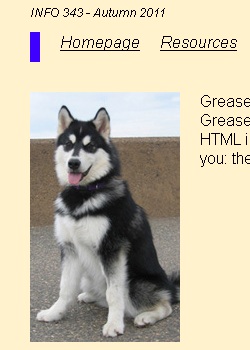
// ==UserScript== // @name pictureOfDubs // @author Terry Brooks // @date June 1, 2011 // @namespace http://www.ischool.washington.edu/ // @include http://projects.ischool.washington.edu/tabrooks/343INFOAutumn11/greaseMonkey/greaseMonkey.htm // @exclude http://faculty.washington.edu/tabrooks/ // ==/UserScript== (function() { try { ////////////////////////////////////////////////////////// // Use Xpath to get image of greasemonkey var myNodeList; myNodeList = document.evaluate( "/html/body/div/div/p[2]/img", document, null, XPathResult.UNORDERED_NODE_SNAPSHOT_TYPE, null); // monkeyNode is img of the greasemonkey var monkeyNode; for (var i = 0; i < myNodeList.snapshotLength; i++) { monkeyNode = myNodeList.snapshotItem(i); } ///////////////////////////////////////////////////////////// // HTTPRequest code GM_xmlhttpRequest({ method: 'GET', url: 'http://www.gohuskies.com/genrel/dubs-about.html', headers: { 'User-agent': 'Mozilla/4.0 (compatible) Greasemonkey', 'Accept': 'text/html', }, onload: function(responseDetails) { // stuff is of string type var stuff = responseDetails.responseText; // Transform string mash page into DOM object var mashPage = document.createElement('div'); mashPage.innerHTML = stuff; // Search mash DOM object with Xpath to the Dawg image var mashNodeList; mashNodeList = document.evaluate( "//table/tbody/tr/td/div[3]/table/tbody/tr/td[2]/img", mashPage, null, XPathResult.UNORDERED_NODE_SNAPSHOT_TYPE, null); // Isolate the Dawg image var dawgNode; for (var i = 0; i < mashNodeList.snapshotLength; i++) { dawgNode = mashNodeList.snapshotItem(i); } // Give the source path of the Dawg image to the greasemonkey image monkeyNode.src = dawgNode.src; } }); ////////////////////////////////////////////////////////////////// } catch (eErr) { alert ("Greasemonkey error: " + eErr); } return; }) ();
How to use Greasemonkey: (1) Use the Firefox browser, (2) Load the Greasemonkey extension (which will require that you restart Firefox), (3) Point your Firefox browser at this webpage, (4) Click on the link below, (5) Firefox will recognize this script as a Greasemonkey script, (6) Click to install this script, (7) Refresh this page. Phyllis Wise should replace the greasemonkey.
Download this script
Do it again! But even faster this time
There is, as you might suspect, more than one way to grease up a web page. The following script is even faster because it contains the complete path to the Phyllis Wise image right in the code.
// ==UserScript== // @name pictureOfDubs2 // @author Terry Brooks // @date June 1, 2011 // @namespace http://www.ischool.washington.edu/ // @include http://projects.ischool.washington.edu/tabrooks/343INFOAutumn11/greaseMonkey/greaseMonkey.htm // @exclude http://faculty.washington.edu/tabrooks/ // ==/UserScript== (function() { try { ////////////////////////////////////////////////////////// // Use Xpath to get image of greasemonkey var myNodeList; myNodeList = document.evaluate( "/html/body/div/div/p[2]/img", document, null, XPathResult.UNORDERED_NODE_SNAPSHOT_TYPE, null); // monkeyNode is img of the greasemonkey var monkeyNode; for (var i = 0; i < myNodeList.snapshotLength; i++) { monkeyNode = myNodeList.snapshotItem(i); } monkeyNode.src = "http://grfx.cstv.com/schools/wash/graphics/auto/dubs-profile.jpg"; } catch (eErr) { alert ("Greasemonkey error: " + eErr); } return; }) ();