Cross-browser problems occur when you wish that your page looks "the same" on every display device, but it looks or acts differently depending on the client device (which varies not only by browser type, version of browser type, but also by type of device -- from hand-held to vast wall screens!)
I'll be brave and tell you flat out right here that I don't have a satisfactory or comprehensive solution to this problem. The following notes are my observations of the strategies that other folks are employing. Good luck!
W3C browser statistics
Limit your support of client device
YUI provides graded browser support, i.e., the most popular browser types and browser versions get first-class support and others receive less support. For example, in April 2011, here is a chart of YUI graded browser support:
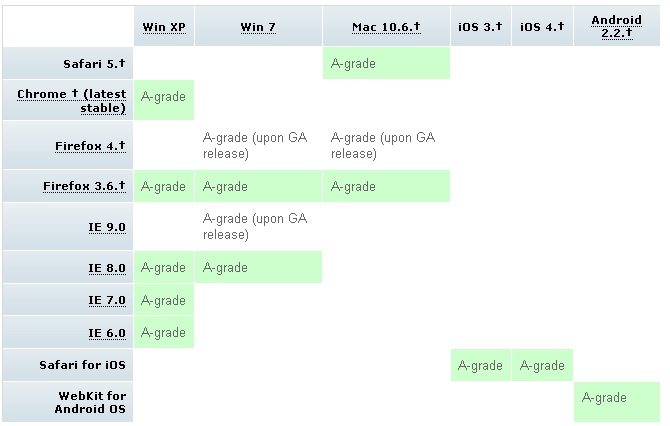
I suppose that the reasonable strategy would be to use a library such as YUI and then rest satisfied that you're serving the greatest number of popular browsers. If you don't want to use a library like YUI, yet you want to use the strategy of graded browser support...well, good luck!
Test for specific support and object detection
Peter Paul Koch, author of Quirksmode, recommends testing for the specific property of interest (usually DOM or JavaScript). For example, read this page and recognize/sympathsize with Peter Paul's frustration. As an example of testing for specific functionality, consider the following piece of Peter Paul's code. Note how he tests which browser is being used before proceeding...
function doSomething(e) { var posx = 0; var posy = 0; if (!e) var e = window.event; if (e.pageX || e.pageY) { posx = e.pageX; posy = e.pageY; } else if (e.clientX || e.clientY) { posx = e.clientX + document.body.scrollLeft + document.documentElement.scrollLeft; posy = e.clientY + document.body.scrollTop + document.documentElement.scrollTop; } // posx and posy contain the mouse position relative to the document // Do something with this information }
Generalizing the above is the idea of object detection. Object detection more or less turns the tables on the strategy of detecting which browser is being used, instead, it focuses on the specific object/functionality that you wish to use and you simply code your way into the use of this object or away from it. Consider the following script that tests for a specific functionality:
<script type="text/javascript"> if (window.addEventListener) { do something that exploits "addEventListener" } else { do something that avoids "addEventListener" } </script>
I imagine that this is a useful strategy to use if the number of objects that you wish to detect remains reasonably small. Testing for hundreds/thousands of objects/functionality might eventually turn into a monster. Consider also the effect of time as browsers grow and develop and eventually all/most browsers have a basic core set of objects and functions. Thus in a few years a test for "addEventListener" might be essentially a waste of time, but your code continues to test for it.
Good luck!
Conditional CSS files
There has been some talk on the Web about using CSS browser selects. This means that you create a CSS file that conditionally styles your page depending on the browser.
Here's the JavaScript that identifies the client browser:
var css_browser_selector = function() {var ua=navigator.userAgent.toLowerCase(), is=function(t){return ua.indexOf(t) != -1;}, h=document.getElementsByTagName('html')[0], b=(!(/opera|webtv/i.test(ua))&&/msie\s(\d)/.test(ua))?('ie ie'+RegExp.$1): is('firefox/2')?'gecko ff2':is('firefox/3')?'gecko ff3': etc., etc.,
Of course, you've have to update this script as browsers change. It also commits you to write big conditional CSS files.
Good luck!
C grade browser list
Yahoo Developers suggests you don't serve these browsers JavaScript or CSS
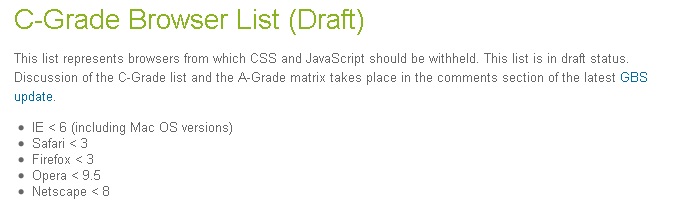
The special case of Internet Explorer
HTML & CSS: The Good Parts Ben Henick (O'Reilly, 2010)
"Internet Explorer 6 is considered a Bad Part unto itself not because it's a burden on the user experience, but instead it's ubiquitous. Where it fails to support something, all web users are denied access to newer web technologies, since most commercial clients will not expend resources on products that can only be enjoyed by a fraction of their intended audience."
Significant shortcomings in Internet Explorer's selector support>
Selector | Earliest supported version |
Notes |
>(direct descendent> | 7 | |
[foo](attribute) | 8 | Simple attribute selectors work in IE 8, but efforts to further narrow their scope fail to show results. |
:first-child, :last-child | 8 | |
:nth-child() | n/a | |
:before | 8 | Counters are ignored |
:after | n/a | |
.foo.bar | 7 | IE6 reads this selector as .bar. |
:hover | 3 | The :hover pseudoclass was only applied to elements other than a as of version 7; in the case of :active, as of version 8 |
hasLayout is an odd property, specific to internet Explorer, that evaluates to true or false when queried via the DOM API. When hasLayout evaluates to true, the applicable element's presentation characteristics are defined somewhere within the rendering engine's logic, rather than being polled. The latter outcome leads to many of the layout bugs that stylists encounter when testing their work product in IE 6.
As it happens, when Microsoft first implemented the box model for Internet Explorer 5/win, it was apparently decided that the standard box model was sub-standard. So, they arranged for the box "width" declaration to refer instead to the outer dimension of the visible bordered box. In other words, in IE, "width" referred to the area within the outer edges of the black borders, but not including the margins (see the above example). The same decision was made for "height," too.
This meant that if the box was given borders and/or padding, the content area (and the whole box) would be smaller when viewed in IE. A major hassle for sure! It affected most every box that was assigned "width" or "height". Even though no height is assigned to our example div, the problem can also affect that dimension.
Fortunately, Microsoft saw the error of its ways and corrected IE's box model in IE6, but only if it is in "standards mode". If it is left in "quirks mode" it still emulates the old IE5.x/win box model. By the way, IE5/mac always obeyed the standard box model.
Thanks to http://www.communitymx.com/content/article.cfm?cid=E0989953B6F20B41
IE 8 is much more standards compliant, but it breaks all those pages out there on the Web with special architecture for earlier versions of IE. Here are some strategies for architecting web pages in the IE community that deal with the different versions of IE.
Good luck!
Making your web site compatible across multiple versions of Internet Explorer
Explains that you need to do the following so that your page works in multiple versions of IE:1. Author your page according to the latest Web standards as supported by Internet Explorer 8 Beta 2. 2. Include a Standards mode DOCTYPE. 3. Use conditional comments to provide fix-ups for legacy versions of Internet Explorer.
Compatibility View Improvements to come in IE8
With IE8's Beta 2 release, we introduced the Compatibility View button. This button enables savvy end-users to resolve compatibility problems they encounter with sites that rely on legacy IE behavior. Specifically, the button enables users to treat specific websites in a non-default way so that they work in the browser. This is a good thing - with Beta 2, a site that relies on IE7 behavior from IE8 but has not specified it explicitly can still work for someone visiting it in IE8.
Despite all the outreach to sites, we saw from the telemetry data that IE8 Beta 2 users still have to use Compatibility View a lot. Looking at our instrumentation, there were high-volume sites like facebook.com, myspace.com, bbc.co.uk, and cnn.com with pages that weren't working for end-users with IE's new standards compliant default. We could also see from our instrumentation that not all IE8 visitors to those sites were clicking the Compatibility View button. So, large groups of people were having a less than great experience because they weren't aware of the manual steps required to make certain sites work.
When users install Windows 7 Beta or the next IE8 update, they get a choice about opting-in to a list of sites that should be displayed in Compatibility View. Sites are on this list based on feedback from other IE8 customers: specifically, for what high-volume sites did other users click the Compatibility View button? This list updates automatically, and helps users who aren't web-savvy have a better experience with web sites that aren't yet IE8-ready.
HTML5, Modernized: Fourth IE9 Platform Preview Available for Developers
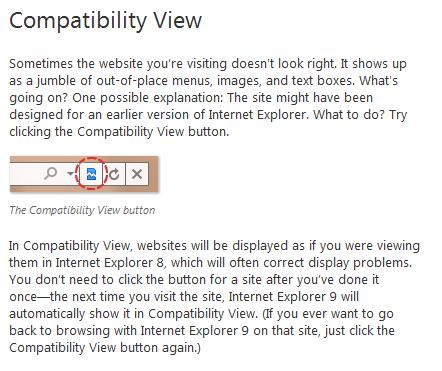
http://windows.microsoft.com/en-US/internet-explorer/products/ie-9/features/compatibility-view