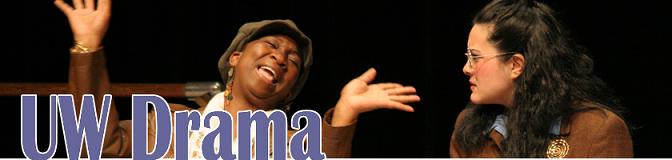
The University of Washington School of Drama is one of this country's leading training institutions for theatre artists and scholars. Seventeen faculty and 17 staff members serve 260 undergraduate majors and 65 graduate students. The School offers a four-year undergraduate liberal arts education, Masters of Fine Arts degrees in acting, design, and directing, and a PhD in theatre history and criticism.
The School of Drama maintains an active performance schedule with plays such as "The American Century" and "The Apollo of Bellac" being performed in Spring 2009.
What they need - and this is where you can help the UW Drama School - is a web widget that would let ticket purchasers slide a couple of date sliders back and forth to capture a span of months, then press a button to see the various plays that the UW School of Drama will be performing.
Here is a screen grab of such a widget.
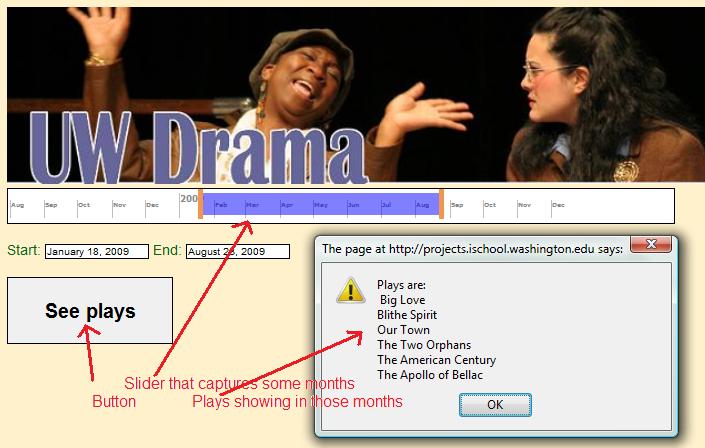
Some development notes
A listing of the plays: playData.js is a JavaScript file that you can include in your project. It lists 22 plays from January 2007 to May 2009. See the fragment below. Note that the plays are constructed as "play" objects.
function play ( ti, au, dir, mon, yr, pl ) { this.title = ti; this.author = au; this.director = dir; this.month = mon; this.year = yr; this.place = pl; } var p1 = new play ( "The Bacchae", "Euripides", "Andrew Tsao", "January", "2007", "Meany Studio" ); var p2 = new play ( "Jane Eyre", "Charlotte Bronte", "Katjana Vadeboncoeur", "February", "2007", "Playhouse" ); ...
Using the "play" objects If you include "playData.js" in your project, you will have all the information about the plays.
<script type="text/javascript" src="playData.js"></script>
You can access the individual play information by addressing the play objects (i.e., "p1" to "p22") by iterating from 1 to 22, constructing the names of the play objects and then evaluating a call to their titles. For example, the following code produces a list of all play titles.
var allPlays = " "; for (var j = 1; j < 23; j++) { var thisTitle = eval("p" + j + ".title"); // This produces: eval(p5.title) allPlays += thisTitle + "\n"; // Play title plus a new line } alert(allPlays);
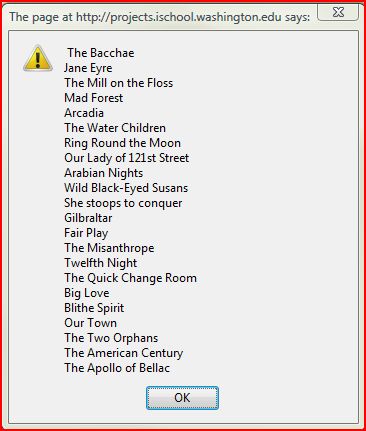
Use the Dateslider widget. Place it directly beneath the photo from the UW Drama School. Create a button for your project. The button drives a function that reads the start date, the end date and then goes through the list of plays and lists the plays that fall within the time limits.
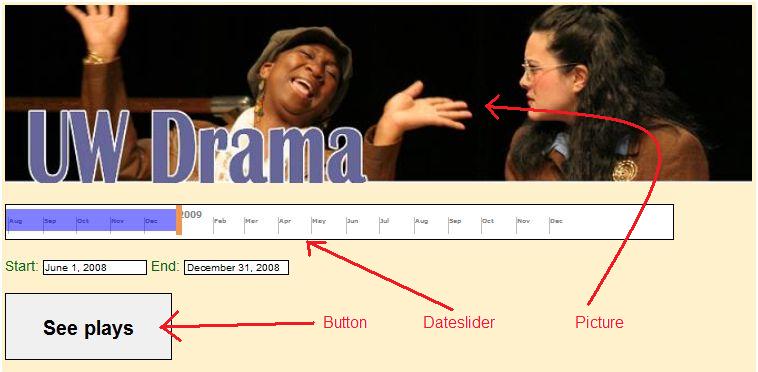
A general strategy Note that the play information in "playData.js" is organized chronologically. I utilized this organization in creating an algorithm for finding plays that fell within the time limits. If I were to construct the beginning and closing dates as numbers, and then construct the play dates as numbers, I can iterate through the play information just once, and find the play dates that occur equal to or greater than the beginning date and occur equal to or less than the closing date. Here's a visualization of such a process:
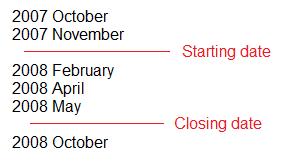
Construct the beginning and closing dates Note that the Dateslider widget displays the start date in an input field with the id "datestart" and the closing date in an input field with the id "dateend". Furthermore, note that Dateslider constructs the dates as "June 1, 2008". This means that if we split that date on whitespace we can easily find the month and year.
// Get the starting date
var getStart = document.getElementById("datestart");
var splitGetStart = getStart.value.split(" "); // Split the date on whitespace
// splitGetStart[0] is the month such as "June"
// splitGetStart[2] is the year such as "2008"
var beginDate = splitGetStart[2] + doMonth(splitGetStart[0]);
The only tricky thing here is that my function doMonth() is used to change the month name (i.e., "June") into a number (i.e., "06").
The function doMonth() is another JavaScript include file. It is merely a Switch function that accepts a Month name and returns a number. Here is a fragment:
function doMonth(monthName) { switch(monthName) { case "January": return "01"; break; case "February": return "02"; break; ...
Selecting the plays You can visit all the play objects by looping from 1 to 23, and for each getting their month and year, and then constructing a single number for the play by adding its year and month.
var thisYear = eval("p" + j + ".year"); // For example: p6.year which is "2007" var thisMonth = eval("p" + j + ".month"); // For example: p6.month which is "September" var thisNumber = thisYear + doMonth(thisMonth); // thisNumber = 200706
Finally, you can compare "thisNumber" to the "beginDate" and "closeDate". Play dates that pass these two conditions fall within the span of months and should be shown to the user of the UW Drama School web site.
if ((thisNumber >= beginDate) && (thisNumber <= closeDate))
Plays that pass through this double condition will be performed within the time limits and should be shown.