Pick A Husky Holiday
Let's get serious for a moment and admit that the Huskies love holidays! The proof of this is easy. Just peruse this page from the Calendar of Religious Holidays and Observances:
Here are the holidays and observances in 2009:
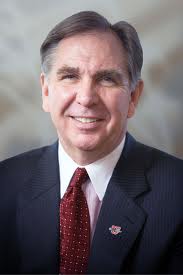
UW President and "Number One Dawg" Michael Young wants you to build a web page widget for the UW holidays and observances!
Furthermore, suppose Mike wants you to use a datepicker widget!
Suppose you visit with President Young in his office and he shows you this sketch of his idea for the holiday widget.
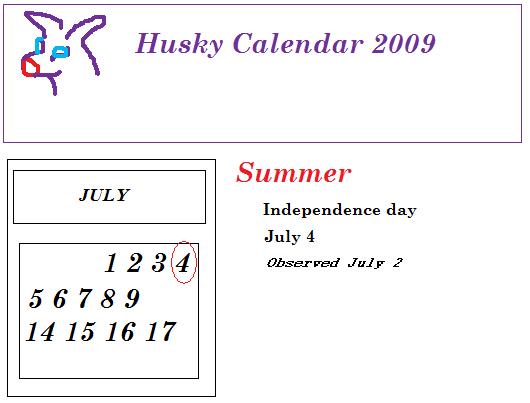
As you can see, Mike has a fairly straight forward visual design in mind...a pretty picture of a Husky dog, the title "Husky Calendar 2009" in purple, the datepicker widget itself in the lower left, and the holiday information shows up in the lower right.
You can find the source information about the 2009 UW holidays and observances above. At ten thousand feet, the project is simple...a person chooses a date in the 2009 datepicker and if the date is a university holiday -- Bango! -- the information about the holiday shows up. On the other hand, if a person chooses a date that is not a holiday, just an bland, but informative, message shows: "Not a holiday".
Some development notes
Separate web page Build your widget on a separate web page.
Choose to work with one date picker widget (not both) date picker widgets.
Handling the chosen date Both the jQuery calendar widget and the Date Picker widget deliver the date as a string where the date elements are separated by a forward slash: "01/15/2009". You can unpack such a well-formed string fairly easily using the split() method and splitting the string on the forward slash.
var i = document.getElementById("heading"); // "dateText" is automatically produced by the jQuery widget ... onSelect: function(dateText) { var i = document.getElementById("heading"); var dateArray = dateText.split("/"); i.innerHTML = "Length:" + dateArray.length + " Month:" + dateArray[0] + " Day:" + dateArray[1] + " Year:" + dateArray[2]; }
![]()
With the Date Picker widget, you can use a button to drive a function to get the date string
function doShow() { var j = document.getElementById("dp-1"); var dateArray = j.value.split("/"); alert("Month: " + dateArray[0] + " Day: " + dateArray[1] + " Year: " + dateArray[2]); }
Architecting the data There are several ways you might architect the data for your widget, but choose a method that permits several holidays during the year to overlap, i.e., June 21 is both the Summer Solstice and Father's Day; April 10 is both Good Friday and falls within Passover, etc. Whatever technique you use will have to permit "June 21", for example, to return more than one holiday.
You might consider defining your own objects in JavaScript. Here's some sample code that creates a new object type, declares a new object of that type and then tests the value of one of the object's properties.
function uwHoliday ( name, number ) { this.givenName = name; this.givenNumber = number; } var tester = new uwHoliday ( "Christmas", "25" ); var num = "25"; function showResults() { if (num == tester.givenNumber) { alert(tester.givenName + " " + tester.givenNumber); } }
![]()
If you do use the strategy of defining your own JavaScript objects, you can add each to an array and then iterate over the array as necessary.
var tester1 = new uwHoliday ( "Christmas", "25" ); var tester2 = new uwHoliday ( "Kwanzaa", "25" ); var tester3 = new uwHoliday ( "Winter solstice", "21"); var allHolidays = new Array(); allHolidays[0] = tester1; allHolidays[1] = tester2; allHolidays[2] = tester3; // inNum comes from a user button such as // <input type="button" value="Press me" onclick="showResults('25')"> function showResults(inNum) { for (var i = 0; i < allHolidays.length; i++) { if ( allHolidays[i].givenNumber == inNum ) { alert(allHolidays[i].givenName + " " + allHolidays[i].givenNumber); } } }
![]()